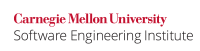
...
The C Standard Annex K functions are still capable of overflowing a buffer if the maximum length of the destination buffer and number of characters to copy are incorrectly specified. ISO/IEC TR 24731 Part II functions can make it more difficult to keep track of memory that must be freed, leading to memory leaks. As a result, the C Standard Annex K and the ISO/IEC TR 24731 Part II functions are not particularly secure but may be useful in preventive maintenance to reduce the likelihood of vulnerabilities in an existing legacy code base.
Noncompliant Code Example
This noncompliant code overflows its buffer if msg
is too long, and it has undefined behavior if msg
is a null pointer:
Code Block | ||||
---|---|---|---|---|
| ||||
void complain(const char *msg) { static const char prefix[] = "Error: "; static const char suffix[] = "\n"; char buf[BUFSIZ]; strcpy(buf, prefix); strcat(buf, msg); strcat(buf, suffix); fputs(buf, stderr); } |
Compliant Solution (Runtime)
This compliant solution will not overflow its buffer:
Code Block | ||||
---|---|---|---|---|
| ||||
void complain(const char *msg) { errno_t err; static const char prefix[] = "Error: "; static const char suffix[] = "\n"; char buf[BUFSIZ]; err = strcpy_s(buf, sizeof(buf), prefix); if (err != 0) { /* Handle error */ } err = strcat_s(buf, sizeof(buf), msg); if (err != 0) { /* Handle error */ } err = strcat_s(buf, sizeof(buf), suffix); if (err != 0) { /* Handle error */ } fputs(buf, stderr); } |
Compliant Solution (Partial Compile Time)
This compliant solution performs some of the checking at compile time using a static assertion. (See DCL03-C. Use a static assertion to test the value of a constant expression.)
Code Block | ||||
---|---|---|---|---|
| ||||
void complain(const char *msg) { errno_t err; static const char prefix[] = "Error: "; static const char suffix[] = "\n"; char buf[BUFSIZ]; /* * Ensure that more than one character * is available for msg */ static_assert(sizeof(buf) > sizeof(prefix) + sizeof(suffix), "Buffer for complain() is too small"); strcpy(buf, prefix); err = strcat_s(buf, sizeof(buf), msg); if (err != 0) { /* Handle error */ } err = strcat_s(buf, sizeof(buf), suffix); if (err != 0) { /* Handle error */ } fputs(buf, stderr); } |
Risk Assessment
String-handling functions defined in the C Standard, subclause 7.24, and elsewhere are susceptible to common programming errors that can lead to serious, exploitable vulnerabilities. Proper use of the C11 Annex K functions can eliminate most of these issues.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
STR07-C | High | Probable | Medium | P12 | L1 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| BADFUNC.BO.OEMTOCHAR BADFUNC.BO.STRCAT BADFUNC.BO.STRCATCHAINW BADFUNC.BO.STRCMP BADFUNC.BO.STRCPY BADFUNC.BO.STRLEN BADFUNC.BO.STRTRNS | Use of OemToAnsi, Use of OemToChar (both include checks for uses of similar functions) Use of strcat (includes checks for uses of similar library functions such as StrCatA(), wcscat(), etc) Use of StrCatChainW Use of strcmp (includes checks for uses of similar library functions such as lstrcmp()) Use of strcpy (includes checks for uses of similar library functions such as StrCCpy(), wcscpy(), etc) Use of strlen (includes checks for uses of similar library functions such as lstrlen()) Use of strtrns | ||||||
|
|
| |||||||
PRQA QA-C |
| Warncall -wc strcpy -wc strcat -wc strncpy -wc strncat | Partially implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
ISO/IEC TR 24731-2:2010 | |
ISO/IEC TR 24772:2013 | Use of Libraries [TRJ] |
Bibliography
[Seacord 2005b] | "Managed String Library for C, C/C++" |
[Seacord 2013] | Chapter 2, "Strings" |
...