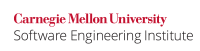
...
In both cases, the function will return some value, but the value returned is not the correct result of the computation.
Many math Domain errors can be prevented by carefully bounds checking the arguments before calling functions, and taking alternative action if the bounds are violated. In particular, the following functions should be bounds checked as follows:
Function | Bounds-checking |
---|---|
-1 <= x && x <= 1 | |
x != 0 || y != 0 | |
x > 0 | |
x > 0 || (x == 0 && y > 0) || (x < 0 && y is an integer) | |
x >= 0 | |
(x == 0) || (x < 0 && x is an integer) || (x is too large) | |
(x == 0) || (x < 0 && x is an integer) || (x is too large) || (x is too small) |
However, for some functions it is not practical to use bounds checking to prevent all errors. In the above pow
example, the bounds check does not prevent the pow(10., 1e6)
range error. In these cases detection must be used, either in addition to bounds checking or instead of bounds checking.
...
Range errors usually can not be prevented, as they are dependent on the implementation of floating-point numbers, as well as the function being applied. Instead of preventing range errors, one should attempt to detect them and take alternative action if a range error occurs.
Wiki Markup |
---|
The following table lists standard mathematical functions, along with any checks that should be performed on their domain, and indicates if they also throw range errors, as reported by \[ISO/IEC 9899:1999\]. If a function has domain checks, one should check its input values, and if a function throws range errors, one should detect if a range error occurs. The standard math functions not on this table, such as {{atan()}} have no domain restrictions and do not throw range errors. |
Function | Domain | Range |
---|---|---|
| -1 <= x && x <= 1 | no |
| x != 0 || y != 0 | no |
| x >= 1 | no |
| -1 < x && x < 1 | no |
none | yes | |
| none | yes |
| none | yes |
| x > 0 | no |
| x > -1 | no |
| x != 0 | yes |
| none | yes |
| none | yes |
x > 0 || (x == 0 && y > 0) || (x < 0 && y is an integer) | yes | |
x >= 0 | no | |
| none | yes |
| (x == 0) || (x < 0 && x is an integer) | yes |
| no | yes |
| y != 0 | no |
| none | yes |
| none | yes |
| none | yes |
Domain Checking
The most reliable way to handle domain errors to prevent them by checking arguments beforehand, as in the following template:
Code Block |
---|
if (/* arguments will cause a domain error */) {
/* handle domain error */
}
else {
/* perform computation */
}
|
Range Checking
Range errors can usually not be prevented, so the most reliable way to handle range errors is to detect when they have occurred and act accordingly. The following approach uses C99 standard functions for floating point errors.
Code Block |
---|
#include <math.h>
#if defined(math_errhandling) \
&& (math_errhandling & MATH_ERREXCEPT)
#include <fenv.h>
#endif
/* ... */
#if defined(math_errhandling) \
&& (math_errhandling & MATH_ERREXCEPT)
feclearexcept(FE_ALL_EXCEPT);
#endif
errno = 0;
/* call the function */
#if !defined(math_errhandling) \
|| (math_errhandling & MATH_ERRNO)
if (errno != 0) {
/* handle range error */
}
#endif
#if defined(math_errhandling) \
&& (math_errhandling & MATH_ERREXCEPT)
if (fetestexcept(FE_INVALID
| FE_DIVBYZERO
| FE_OVERFLOW
| FE_UNDERFLOW) != 0)
|
...
acos(x), asin(x)
Noncompliant Code Example
The following noncompliant code computes the arc cosine of the variable x
Code Block | ||
---|---|---|
| ||
double x;
double result;
/* Set the value for x */
result = acos(x);
|
Wiki Markup |
---|
However, this code may produce a _domain error_ if {{x}} is not in the range \[-1, \+1\]. |
Compliant Solution
The following compliant solution uses bounds checking to ensure that there is not a domain error.
Code Block | ||
---|---|---|
| ||
double x;
double result;
/* Set the value for x */
if ( isnan(x) || isless(x,-1) || isgreater(x, 1) ){
/* handle domain error */
}
result = acos(x);
|
...
atan2(y, x)
Noncompliant Code Example
The following noncompliant code computes the arc tangent of the two variables x
and y
.
Code Block | ||
---|---|---|
| ||
double x;
double y;
double result;
/* Set the value for x and y */
result = atan2(y, x);
|
However, this code may produce a domain error if both x
and y
are zero.
Compliant Solution
The following compliant solution tests the arguments to ensure that there is not a domain error.
Code Block | ||
---|---|---|
| ||
double x;
double y;
double result;
/* Set the value for x and y */
if ( (x == 0.f) && (y == 0.f) ) {
/* handle domain error */
}
result = atan2(y, x);
|
...
log(x), log10(x)
Noncompliant Code Example
The following noncompliant code determines the natural logarithm of x
.
Code Block | ||
---|---|---|
| ||
double x;
double result;
/* Set the value for x */
result = log(x);
|
However, this code may produce a domain error if x
is negative and a range error if x
is zero.
Compliant Solution
The following compliant solution tests the suspect arguments to ensure that no domain errors or range errors are raised.
Code Block | ||
---|---|---|
| ||
double x; double result; /* Set the value for x */ if (isnan(x) || islessequal(x, 0)) { /* handle domain and range errorsrange error */ } result = log(x); |
...
pow(x, y)
Mathematically, the domain of pow( x, y)
dictates that if x
is 0, then y
must be strictly positive, and if x
is negative, then y
must be an integer. However, because pow()
can yield numbers of very large magnitude or very small magnitude, the set of inputs that do not cause a range error is not only more limited, but poorly defined.
Noncompliant Code Example
The following noncompliant code raises x
to the power of y
.
Code Block | ||
---|---|---|
| ||
double x;
double y;
double result;
result = pow(x, y);
|
However, this code may produce a domain error if x
is negative and y
is not an integer, or if x
is zero and y
is zero. A domain error or range error may occur if x
is zero and y
is negative, and a range error may occur if the result cannot be represented as a double
.
Noncompliant Code Example
This noncompliant code example only performs bounds checking on x
and y
. It prevents domain errors and some range errors, but does not prevent range errors where the result cannot be represented as a double
(see the Error Checking and Detection section below regarding ways to mitigate the effects of a range error).
Code Block | ||
---|---|---|
| ||
double x;
double y;
double result;
if (((x == 0.f) && islessequal(y, 0)) ||
(isless(x, 0))) {
/* handle domain and range errors */
}
result = pow(x, y);
|
...
sqrt(x)
Noncompliant Code Example
The following noncompliant code determines the square root of x
Code Block | ||
---|---|---|
| ||
double x;
double result;
result = sqrt(x);
|
However, this code may produce a domain error if x
is negative.
Compliant Solution
The following compliant solution tests the suspect argument to ensure that no domain error is raised.
Code Block | ||
---|---|---|
| ||
double x;
double result;
if (isless(x, 0)){
/* handle domain error */
}
result = sqrt(x);
|
...
lgamma(x), tgamma(x)
Mathematically speaking, the domain of both lgamma()
and tgamma()
is the set of real numbers excepting the non-positive integers. However, since both functions often yield numbers of very large magnitude or very small magnitude, the set of inputs that do not cause a range error is not only more limited, but poorly defined. For instance, tgamma(-90.5)
is close enough to 0 that it causes an underflow error on 64-bit IEEE double
implementations.
Noncompliant Code Example
The following noncompliant code example computes the gamma value of x
.
Code Block | ||
---|---|---|
| ||
double x;
double d = tgamma(x);
|
However, this code may produce a domain error if x
is 0 or a negative integer. Also, a range error may occur if the result cannot be represented as a double
.
Noncompliant Code Example
This noncompliant code example attempts to prevent domain errors, but does not prevent range errors. The result may be an underflow or overflow error. See the Error Checking and Detection section below regarding ways to mitigate the effects of a range error.
Code Block | ||
---|---|---|
| ||
double x;
if ((x == 0) || (x < 0 && x == nearbyint(x))) {
/* handle error */
}
double d = tgamma(x);
|
...
#endif
|
See FLP03-C. Detect and handle floating point errors for more details on how to detect floating point errors.
Anchor | ||||
---|---|---|---|---|
|
Error Checking and Detection
Wiki Markup |
---|
The exact treatment of error conditions from math functions is quite complicated. C99 Section 7.12.1 defines the following behavior for floating point overflow \[[ISO/IEC 9899:1999|AA. C References#ISO/IEC 9899-1999]\] |
A floating result overflows if the magnitude of the mathematical result is finite but so large that the mathematical result cannot be represented without extraordinary roundoff error in an object of the specified type. If a floating result overflows and default rounding is in effect, or if the mathematical result is an exact infinity from finite arguments (for example
log(0.0)
), then the function returns the value of the macroHUGE_VAL
,HUGE_VALF
, orHUGE_VALL
according to the return type, with the same sign as the correct value of the function; if the integer expressionmath_errhandling & MATH_ERRNO
is nonzero, the integer expressionerrno
acquires the valueERANGE
; if the integer expressionmath_errhandling & MATH_ERREXCEPT
is nonzero, the ''divide-by-zero'' floating-point exception is raised if the mathematical result is an exact infinity and the ''overflow'' floating-point exception is raised otherwise.
It is best not to check for errors by comparing the returned value against HUGE_VAL
or 0
for several reasons:
- These are in general valid (albeit unlikely) data values.
- Making such tests requires detailed knowledge of the various error returns for each math function.
- There are three different possibilities,
-HUGE_VAL
,0
, andHUGE_VAL
, and you must know which are possible in each case. - Different versions of the library have differed in their error-return behavior.
Wiki Markup |
---|
It is also difficult to check for math errors using {{errno}} because an implementation might not set it. For real functions, the programmer can tell whether the implementation sets {{errno}} by checking whether {{math_errhandling & MATH_ERRNO}} is nonzero. For complex functions, the C99 Section 7.3.2 simply states "an implementation may set {{errno}} but is not required to" \[[ISO/IEC 9899:1999|AA. C References#ISO/IEC 9899-1999]\]. |
Implementation Details
System V Interface Definition, Third Edition
The System V Interface Definition, Third Edition (SVID3) provides more control over the treatment of errors in the math library. The user can provide a function named matherr
that is invoked if errors occur in a math function. This function can print diagnostics, terminate the execution, or specify the desired return-value. The matherr()
function has not been adopted by C99, so its use is not generally portable.
Anchor | ||||
---|---|---|---|---|
|
sqrt(x)
Noncompliant Code Example
The following noncompliant code determines the square root of x
Code Block | ||
---|---|---|
| ||
double x;
double result;
result = sqrt(x);
|
However, this code may produce a domain error if x
is negative.
Compliant Solution
Since this function has domain errors but no range errors, one can use bounds checking to prevent domain errors.
Code Block | ||
---|---|---|
| ||
double x;
double result;
if (isless(x, 0)) {
/* handle domain error */
}
result = sqrt(x);
|
Anchor | ||||
---|---|---|---|---|
|
cosh
, sinh
Noncompliant Code Example
The following noncompliant code determines the hyperbolic cosine of x
.
Code Block | ||
---|---|---|
| ||
double x;
double result;
result = cosh(x);
|
However, this code may produce a range error if x
has a very large magnitude.
Compliant Solution
Since this function has no domain errors but may have range errors, one must detect a range error and act accordingly.
Code Block | ||
---|---|---|
| ||
#include <math.h>
#if defined(math_errhandling) \
&& (math_errhandling & MATH_ERREXCEPT)
#include <fenv.h>
#endif
/* ... */
#if defined(math_errhandling) \
&& (math_errhandling & MATH_ERREXCEPT)
feclearexcept(FE_ALL_EXCEPT);
#endif
errno = 0;
double x;
double result;
result = sinh(x);
#if !defined(math_errhandling) \
|| (math_errhandling & MATH_ERRNO)
if (errno != 0) {
/* handle range error */
}
#endif
#if defined(math_errhandling) \
&& (math_errhandling & MATH_ERREXCEPT)
if (fetestexcept(FE_INVALID
| FE_DIVBYZERO
| FE_OVERFLOW
| FE_UNDERFLOW) != 0)
{
/* handle range error */
}
#endif
|
Anchor | ||||
---|---|---|---|---|
|
pow(x, y)
Noncompliant Code Example
The following noncompliant code raises x
to the power of y
.
Code Block | ||
---|---|---|
| ||
double x;
double y;
double result;
result = pow(x, y);
|
However, this code may produce a domain error if x
is negative and y
is not an integer, or if x
is zero and y
is zero. A domain error or range error may occur if x
is zero and y
is negative, and a range error may occur if the result cannot be represented as a double
.
Noncompliant Code Example
Since the pow()
function can produce both domain errors and range errors, we must first check that x
and y
lie within the proper domain. We must also detect if a range error occurs, and act accordingly
...
Error Checking and Detection
Wiki Markup |
---|
The exact treatment of error conditions from math functions is quite complicated. C99 Section 7.12.1 defines the following behavior for floating point overflow \[[ISO/IEC 9899:1999|AA. C References#ISO/IEC 9899-1999]\] |
A floating result overflows if the magnitude of the mathematical result is finite but so large that the mathematical result cannot be represented without extraordinary roundoff error in an object of the specified type. If a floating result overflows and default rounding is in effect, or if the mathematical result is an exact infinity from finite arguments (for example
log(0.0)
), then the function returns the value of the macroHUGE_VAL
,HUGE_VALF
, orHUGE_VALL
according to the return type, with the same sign as the correct value of the function; if the integer expressionmath_errhandling & MATH_ERRNO
is nonzero, the integer expressionerrno
acquires the valueERANGE
; if the integer expressionmath_errhandling & MATH_ERREXCEPT
is nonzero, the ''divide-by-zero'' floating-point exception is raised if the mathematical result is an exact infinity and the ''overflow'' floating-point exception is raised otherwise.
It is best not to check for errors by comparing the returned value against HUGE_VAL
or 0
for several reasons:
- These are in general valid (albeit unlikely) data values.
- Making such tests requires detailed knowledge of the various error returns for each math function.
- There are three different possibilities,
-HUGE_VAL
,0
, andHUGE_VAL
, and you must know which are possible in each case. - Different versions of the library have differed in their error-return behavior.
Wiki Markup |
---|
It is also difficult to check for math errors using {{errno}} because an implementation might not set it. For real functions, the programmer can tell whether the implementation sets {{errno}} by checking whether {{math_errhandling & MATH_ERRNO}} is nonzero. For complex functions, the C99 Section 7.3.2 simply states "an implementation may set {{errno}} but is not required to" \[[ISO/IEC 9899:1999|AA. C References#ISO/IEC 9899-1999]\]. |
Compliant Solution (Error Checking)
The most reliable way to test for errors is by checking arguments beforehand, as in the following compliant solution:
Code Block | ||
---|---|---|
| ||
if (/* arguments will cause a domain or range error */) {
/* handle the error */
}
else {
/* perform computation */
}
|
For functions where argument validation is difficult, including pow()
, erfc()
, lgamma()
, and tgamma()
, one can employ the following approach. This approach uses C99 standard functions for floating point errors.
Code Block | ||
---|---|---|
| ||
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; /* call the function */ #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0)double x; double y; double result; if (((x == 0.f) && islessequal(y, 0)) || (isless(x, 0))) { /* handle domain error */ } #endif result = pow(x, y); #if !defined(math_errhandling) \ &&|| (math_errhandling & MATH_ERREXCEPTERRNO) if (fetestexcept(FE_INVALID | FE_DIVBYZEROerrno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_OVERFLOWDIVBYZERO | FE_UNDERFLOW) != 0) { /* handle error */ } #endif |
See FLP03-C. Detect and handle floating point errors for more details on how to detect floating point errors.
Implementation Details
System V Interface Definition, Third Edition
...
OVERFLOW
| FE_UNDERFLOW) != 0)
{
/* handle range error */
}
#endif
|
Risk Assessment
Failure to properly verify arguments supplied to math functions may result in unexpected results.
...