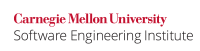
...
Code Block | ||
---|---|---|
| ||
void foo(const int * x) { if (x != NULL) { *x = 3; /* generates compiler warningerror */ } /* ... */ } |
Compliant Solution
...
Code Block | ||
---|---|---|
| ||
char *strcat_nc(char *s1, char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[] = "str3"; const char str4[] = "str4"; strcat_nc(str3, str2); /* Compiler warns that str2 is const */ strcat_nc(str1, str3); /* Oops, attempts to overwrite string literal! */ strcat_nc(str4, str3); /* Compiler warns that str4 is const */ |
The function would behave the same as strcat()
, but the compiler generates warnings in incorrect locations, and fails to generate them in correct locations.
...
Code Block | ||
---|---|---|
| ||
char *strcat(char *s1, const char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[] = "str3"; const char str4[] = "str4"; strcat(str3, str2); strcat(str1str3, str3str1); /* Note:Args reversed argsto prevent overwriting string literal */ strcat(str4, str3); /* different 'const' qualifiers Compiler warns that str4 is const */ |
The const
-qualification of the second argument s2
eliminates the spurious warning in the initial invocation, but maintains the valid warning on the final invocation in which a const
-qualified object is passed as the first argument (which can change). Finally, the middle strcat()
invocation is now valid, as str1
is a valid destination string, as the string exists on the stack and may be safely modified.
...