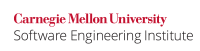
...
This noncompliant code example leads to undefined behavior if the integral part of f1
f_a
cannot be represented as an integer:
Code Block | ||||
---|---|---|---|---|
| ||||
float f1f_a; int i1i_a; /* initialize flfloat f_a */ i1i_a = f1f_a; /* Undefined if the integral part of f1f_a > INT_MAX */ |
Compliant Solution (Int-Float)
...
Code Block | ||||
---|---|---|---|---|
| ||||
float f1f_a; int i1i_a; /* initialize flfloat f_a */ if (f1f_a > (float) INT_MAX || f1f_a < (float) INT_MIN) { /* Handle error */ } else { i1i_a = f1f_a; } |
Noncompliant Code Example (Demotions)
...
Code Block | ||||
---|---|---|---|---|
| ||||
long double ldbig_d; double d1d_a; double d2d_b; float f1f_a; float f2f_b; /* initializations */ f1f_a = (float)d1d_a; f2f_b = (float)ldbig_d; d2d_b = (double)ldbig_d; |
As a result of these conversions, it is possible that d1
d_a
is outside the range of values that can be represented by a float or that ld
big_d
is outside the range of values that can be represented as either a float
or a double
. If this is the case, the result is undefined.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <float.h> long double ldbig_d; double d1d_a; double d2d_b; float f1f_a; float f2f_b; /* initializations */ if (d1d_a > FLT_MAX || d1d_a < -FLT_MAX) { /* Handle error condition */ } else { f1f_a = (float)d1d_a; } if (ldbig_d > FLT_MAX || ldbig_d < -FLT_MAX) { /* Handle error condition */ } else { f2f_b = (float)ldbig_d; } if (ldbig_d > DBL_MAX || ldbig_d < -DBL_MAX) { /* Handle error condition */ } else { d2d_b = (double)ldbig_d; } |
Risk Assessment
Failing to check that a floating-point value fits within a demoted type can result in a value too large to be represented by the new type, resulting in undefined behavior.
...