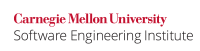
This standard recommends the inclusion of diagnostic tests into your program using the assert()
macro or other mechanisms (see MSC11-A. Incorporate diagnostic tests using assertions). Static assertion is a new facility in the C++ 0X draft standard. This facility gives the ability to make assertions enables assertions to be made at compile time rather than runtime, providing the following advantages:
- all All processing must be performed during compile time – - no runtime cost in space or time is tolerable.
- assertion Assertion failure must result in a meaningful and informative diagnostic error message.
- it It can be used at file or block scope.
- misuse Misuse does not result in silent malfunction , but rather is diagnosed at compile time.
Static assertions take the form:
Code Block |
---|
static_assert(constant-expression, string-literal); |
In a static assert declaration, the constant-expression
is a constant expression that can be contextually converted to bool
. If the value of the expression when converted is true, the declaration has no effect. Otherwise the program is ill-formed, and a diagnostic message (which includes the text of the string-literal
) is issued at compile time. For example:
...
For assertions involving only constant expressions, some implementations allow the use of a preprocessor conditional statement, as in this example:
Code Block | ||
---|---|---|
| ||
struct timer { uint8_t MODE; uint32_t DATA; uint32_t COUNT; }; #if (offsetof(timer, DATA) != 4) #error DATA must be at offset 4 #endif |
...
Unfortunately, this solution is not portable. C99 does not require that implementations support sizeof
, offsetof
, or enumeration constants in #if
conditions. According to Section 6.10.1, "Conditional inclusion," , all identifiers in the expression that controls conditional inclusion either are , or are not , macro names. Some compilers allow these constructs in conditionals as an extension, but most do not.
...
The static_assert()
macro accepts a constant expression e
, which is evaluated as the first operand to the conditional operator. If e
evaluates to nonzero, an array type with a dimension of 1 is defined; otherwise an array type with a dimension of -1 is defined. Because it is invalid to declare an array with a negative dimension, the resulting type definition will be flagged by the compiler. The name of the array is used to indicate the location of the failed assertion.
The macro argument string-literal
is ignored in this case, ; this is meant for future compatibility.
Wiki Markup |
---|
The {{JOIN()}} macro used the {{\##}} operator \[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\] to concatenate tokens. See \[[PRE05-A. Understand macro replacement when concatenating tokens]\] to understand how macro replacement behaves in C when using the {{\##}} operator. |
...
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...
Wiki Markup |
---|
\[[Eckel 07|AA. C References#Eckel 07]\] \[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\] Section 6.10.1, "Conditional inclusion," and Section 6.10.3.3, "The ## operator" \[[Klarer 04|AA. C References#Klarer 04]\] \[[Saks 05|AA. C References#Saks 05]\] \[[Saks 08|AA. C References#Saks 08]\] |
...
DCL02-A. Use visually distinct identifiers 02. Declarations and Initialization (DCL) DCL04-A. Take care when declaring more than one variable per declaration