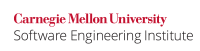
...
This example from Brian Kernighan and Dennis Ritchie [Kernighan 1988] shows both the incorrect and correct techniques for deleting items from freeing the memory associated with a linked list. The incorrect solution, clearly marked as wrong in their book, is bad because In their incorrect solution, p
is freed before the p->next
is executed, so p->next
reads memory that has already been freed.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> struct node { int value; struct node *next; }; void funcfree_list(struct node *head) { for (struct node *p = head; p != NULL; p = p->next) { free(p); } } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> struct node { int value; struct node *next; }; void funcfree_list(struct node *head) { struct node *q; for (struct node *p = head; p != NULL; p = q) { q = p->next; free(p); } } |
...