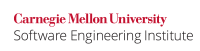
...
However, the random()
function uses time(0)
as seed. With a trivial seed like time(0)
, however, the results from random()
are also predictable.
Compliant Solution (Linux, Solaris, Mac OS X, NetBSD, OpenBSD)
To generate an unpredictable number, use an unpredictable seed and a cryptographically strong mixing function. On Unix systems, for example, decent results can be obtained by reading /dev/urandom
, which will not block the application.
...
The rand48
family of functions provides another psuedo-random alternative.
Code Block | ||
---|---|---|
| ||
unsigned long int li;
FILE* fd;
if(!(fd = fopen("/dev/random", "r")) {
/* Handle error condition */
}
if(fread(&li, sizeof(li), 1, fd) != sizeof(li)) {
/* Handle error condition */
}
fclose(fd);
printf("Random number: %lu\n", li);
|
Compliant Solution (Windows)
...
(someone on a Windows machine should test this out - avolkovi)
...
On Windows platforms, the CryptGenRandom()
function may be used to generate cryptographically strong random numbers. It is important to note, however, that the exact details of the implementation are unknown, and it is undetermined as to what source of entropy the CryptGenRandom()
uses.
If an application has access to a good random source, it can fill the
pbBuffer
buffer with some random data before callingCryptGenRandom()
. The CSP then uses this data to further randomize its internal seed. It is acceptable to omit the step of initializing thepbBuffer
buffer before callingCryptGenRandom()
.
Code Block | ||
---|---|---|
| ||
HCRYPTPROV hCryptProv;
union {
BYTE bs[sizeof(long int)];
long int li;
} rand_buf;
if(!CryptGenRandom(hCryptProv, sizeof(rand_buf), &rand_buf) {
/* Handle error */
} else {
printf("Random number: %lu\n", rand_buf.li);
}
|
Risk Assessment
Using the rand()
function leads to possibly predictable random numbers.
...
Wiki Markup |
---|
\[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\] Section 7.20.2.1, "The rand function" |
...