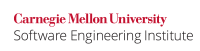
...
Code Block | ||
---|---|---|
| ||
int do_work(int seconds_to_work) { time_t start = time(0NULL); if (start == (time_t)(-1)) { /* Handle error */ } while (time(NULL) < start + seconds_to_work) { do_some_work();/* ... */ } } |
Compliant Solution
This compliant solution uses difftime()
to determine the difference between two time_t
values. difftime()
returns the number of seconds from the second parameter until the first parameter and returns the result as a double
.
Code Block | ||
---|---|---|
| ||
int do_work(int seconds_to_work) { time_t start = time(0NULL); time_t current = start; if (start == (time_t)(-1)) { /* Handle error */ } while (difftime(current, start) < seconds_to_work) { current = time(0NULL); if (current == (time_t)(-1)) { /* Handle error */ } /* do_some_work();... */ } } |
Note that this loop may still not exit, because the range of time_t
may not be able to represent two times seconds_to_work
apart.
...