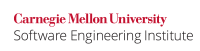
Wiki Markup |
---|
The size of a structure is not always equal to the sum of the sizes of its members. According to Section 6.7.2.1 of the C99 standard, "There may be unnamed padding within a structure object, but not at its beginning." \[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\]. |
...
Padding is also referred to as "Struct Member Alignment." . Many compilers provide a flag that controls how the members of a structure are packed into memory. Modifying this flag may cause the size of the structures to vary. Most compilers also include a keyword that removes all padding; the resulting structures are referred to as packed structures.
...
Wiki Markup |
---|
This non-compliant code example assumes that the size of {{struct buffer}} is equal to the {{sizeof(size_t) + (sizeof(char) * 50)}}, which may not be the case \[[Dowd 06|AA. C References#Dowd 06]\]. The size of {{struct buffer}} may actually be a larger due to structure padding. |
Code Block | ||
---|---|---|
| ||
struct buffer { size_t size; char bufferC[50]; }; /* ... */ void func(struct buffer *buf) { /* assuming sizeof(size_t) is 4, sizeof(size_t)+sizeof(char)*50 equals 54 */ struct buffer *buf_cpy = malloc(sizeof(size_t)+(sizeof(char)*50)); if (buf_cpy == NULL) { /* Handle malloc() error */ } /* * with padding, sizeof(struct buffer) may be greater than 54, causing * some data to be written outside the bounds of the memory allocated */ memcpy(buf_cpy, buf, sizeof(struct buffer)); /* ... */ free(buf_cpy); } |
Compliant Solution
Accounting for structure padding prevents these types of errors.
Code Block | ||
---|---|---|
| ||
struct buffer { size_t size; char bufferC[50]; }; /* ... */ void func(struct buffer *buf) { struct buffer *buf_cpy = malloc(sizeof(struct buffer)); if (buf_cpy == NULL) { /* Handle malloc() error */ } /* ... */ memcpy(buf_cpy, buf, sizeof(struct buffer)); /* ... */ free(buf_cpy); } |
Risk Assessment
Failure to correctly determine the size of a structure can lead to subtle logic errors and incorrect calculations.
...
Wiki Markup |
---|
\[[Dowd 06|AA. C References#Dowd 06]\] Chapter 6, "C Language Issues" (Structure Padding 284-287)
\[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\] Section 6.7.2.1, "Structure and union specifiers"
\[[Sloss 04|AA. C References#Sloss 04]\] Section 5.7, "Structure Arrangement" |
...
EXP02-A. The second operands of the logical AND and OR operators should not contain side effects 03. Expressions (EXP) EXP04-A. Do not perform byte-by-byte comparisons between structures