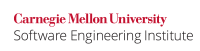
...
- size of a bit-field member of a structure
- size of an array (except in the case of variable length arrays)
- value of an enumeration constant
- value of a
case
constant.
If any of these are required, then an integer constant (an rvalue) must be used. For integer constants, it is preferable to use an enum
instead of a const
-qualified object as this eliminates the possibility of taking the address of the integer constant and does not required that storage is allocated for the value.
Non-Compliant Code
...
(object-like macro)
A preprocessing directive of the form:
#
define
identifier replacement-list new-line
Wiki Markup |
---|
defines an _object-like_ macro that causes each subsequent instance of the macro name to be replaced by the replacement list of preprocessing tokens that constitute the remainder of the directive \[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\]. |
In this non-compliant code In this example, PI
is defined using a as an object-like macro. In Following the codedefinition, the value is introduced each subsequent occurrence of the string "PI" is replaced by the string "3.14159" by textual substitution.
Code Block | ||
---|---|---|
| ||
#define PI 3.14159 /* ... */ float degrees; float radians; /* ... */ radians = degrees * PI / 180; |
An unsuffixed floating constant, as in this example, has type double
. If suffixed by the letter f
or F
, it has type float
. If suffixed by the letter l
or L
, it has type long double
.
Compliant Solution
...
In this compliant solution, the constant pi
is defined declared as a const
variable-qualified object.
Code Block | ||
---|---|---|
| ||
float const pi = 3.14159; /* ... */ float degrees; float radians; /* ... */ radians = degrees * pi / 180; |
This is the best solution for non-integer constants.
Non-Compliant Code Example
...
(immutable integer values)
In this non-compliant code example, max
is declared as a const
-qualified object. While declaring non-integer constants as const
-qualified object is the best that can be done in C, for integer constants we can do better. Declaring Delcaring immutable integer values as const
-qualified objects still allows the programmer to take the address of the object. Also, the constant const
-qualified integers cannot be used in locations where an integer constant is required, such as the size of an arrayvalue of a case
constant.
Code Block | ||
---|---|---|
| ||
int const max = 15; int a[max]; /* invalid declaration outside of a function */ int const *p; p = &max; /* legal to take the address of a const-qualified object */ |
Most C compilers will also allocate memory for the const
-qualified objectobjects.
Compliant Solution
...
(enum)
This compliant solution uses declares max
as an enum
rather than a const
-qualified object or a macro definition.
...