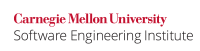
...
This code example is noncompliant because the string referenced by tmpvar
may be overwritten as a result of the second call to the getenv()
function. As a result, it is possible that both tmpvar
and tempvar
will compare equal even if the two environment variables have different values.
Compliant Solution
This compliant solution uses only the C malloc()
and strcpy()
functions to copy the string returned by getenv()
into a dynamically allocated buffer:
Code Block | ||||
---|---|---|---|---|
| ||||
char *tmpvar;
char *tempvar;
const char *temp = getenv("TMP");
if (temp != NULL) {
tmpvar = (char *)malloc(strlen(temp)+1);
if (tmpvar != NULL) {
strcpy(tmpvar, temp);
} else {
/* Handle error */
}
} else {
/* Handle error */
}
temp = getenv("TEMP");
if (temp != NULL) {
tempvar = (char *)malloc(strlen(temp)+1);
if (tempvar != NULL) {
strcpy(tempvar, temp);
} else {
/* Handle error */
}
} else {
/* Handle error */
}
if (strcmp(tmpvar, tempvar) == 0) {
printf("TMP and TEMP are the same.\n");
} else {
printf("TMP and TEMP are NOT the same.\n");
}
free(tmpvar);
tmpvar = NULL;
free(tempvar);
tempvar = NULL;
|
Compliant Solution (C11 Annex K)
C11 Annex K provides the getenv_s()
function for getting a value from the current environment [ISO/IEC 9899:2011]. However, note that according to the standard, getenv_s()
can still have data races with other threads of execution which modify the environment list.
...
Code Block | ||||
---|---|---|---|---|
| ||||
char *tmpvar;
char *tempvar;
const char *temp = getenv("TMP");
if (temp != NULL) {
tmpvar = strdup(temp);
if (tmpvar == NULL) {
/* Handle error */
}
} else {
/* Handle error */
}
temp = getenv("TEMP");
if (temp != NULL) {
tempvar = strdup(temp);
if (tempvar == NULL) {
/* Handle error */
}
} else {
/* Handle error */
}
if (strcmp(tmpvar, tempvar) == 0) {
printf("TMP and TEMP are the same.\n");
} else {
printf("TMP and TEMP are NOT the same.\n");
}
free(tmpvar);
tmpvar = NULL;
free(tempvar);
tempvar = NULL;
|
Compliant Solution
This compliant solution uses only the C malloc()
and strcpy()
functions to copy the string returned by getenv()
into a dynamically allocated buffer:
Code Block | ||||
---|---|---|---|---|
| ||||
char *tmpvar; char *tempvar; const char *temp = getenv("TMP"); if (temp != NULL) { tmpvar = (char *)malloc(strlen(temp)+1); if (tmpvar != NULL) { strcpy(tmpvar, temp); } else { /* Handle error */ } } else { /* Handle error */ } temp = getenv("TEMP"); if (temp != NULL) { tempvar = (char *)malloc(strlen(temp)+1); if (tempvar != NULL) { strcpy(tempvar, temp); } else { /* Handle error */ } } else { /* Handle error */ } if (strcmp(tmpvar, tempvar) == 0) { printf("TMP and TEMP are the same.\n"); } else { printf("TMP and TEMP are NOT the same.\n"); } free(tmpvar); tmpvar = NULL; free(tempvar); tempvar = NULL; |
Risk Assessment
Storing the pointer to the string returned by getenv()
can result in overwritten environmental data.
...