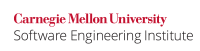
...
According to the C Standard, the behavior of a program that uses the value of a pointer that refers to space deallocated by a call to the free()
or realloc()
function is undefined. (See undefined behavior 177 of Annex J.) Similarly, if an object is referred to outside of its lifetime, the behavior is undefined. (See undefined behavior 9 of Annex J.)
Reading a pointer to deallocated memory is undefined behavior because the pointer value is indeterminate and can have a trap representation. In the latter case, doing so may cause a hardware trap.
...
Code Block | ||||
---|---|---|---|---|
| ||||
void gdClipSetAdd(gdImagePtr im,gdClipRectanglePtr rect) {
gdClipRectanglePtr more;
if (im->clip == 0) {
/* ... */
}
if (im->clip->count == im->clip->max) {
more = gdRealloc (im->clip->list,(im->clip->max + 8) *
sizeof (gdClipRectangle));
if (more == 0) return;
im->clip->max += 8;
im->clip->list = more;
}
im->clip->list[im->clip->count] = (*rect);
im->clip->count++;
|
Noncompliant Code Example
In this example, an object is referred to outside of its lifetime:
Code Block | ||||
---|---|---|---|---|
| ||||
int *get_ptr(void) {
int obj = 12;
return &obj;
}
void func(void) {
int *ptr = get_ptr();
*ptr = 42;
} |
Compliant Solution
In this compliant solution, allocated storage is used instead of automatic storage for the pointer:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> int *get_ptr(void) { int *ptr = (int *)malloc(sizeof(int)); if (!ptr) { return 0; } *ptr = 12; return ptr; } void func(void) { int *ptr = get_ptr(); if (ptr) { *ptr = 42; free(ptr); ptr = 0; } } |
Risk Assessment
Reading memory that has already been freed can lead to abnormal program termination and denial-of-service attacks. Writing memory that has already been freed can lead to the execution of arbitrary code with the permissions of the vulnerable process.
...