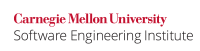
...
Code Block | ||
---|---|---|
| ||
long long a = 1; const char msg[] = "Default message"; /* ... */ printf("%lld %s", a, msg); |
Noncompliant Code Example (NULL Error)
C99, Section 6.3.2.3 (Pointers) says:
An integer constant expression with the value 0, or such an expression cast to type
void *, is called a null pointer constant.55) If a null pointer constant is converted to a
pointer type, the resulting pointer, called a null pointer, is guaranteed to compare unequal
to a pointer to any object or function....
The macro NULL is defined in <stddef.h> (and other headers) as a null pointer constant; see 7.17.
Because C99 allows NULL to be either an integer constant or a pointer constant, any architecture where integers are not the same size as pointers (such as LP64) might present a particular vulnerability with variadic functions. If NULL is defined as an integer on such a platform, then sizeof(NULL) != sizeof(void*)
. Consequently variadic functions that take a pointer type will not correctly promote NULL, leading to undefined behavior. Consequently, the following code may have undefined behavior:
Code Block | ||
---|---|---|
| ||
printf("%p %d\n", NULL, 1);
|
On a LP64 system, this code example might interpret NULL as high-order bits with the following number 1 as low-order bits, and consequently print a pointer with the value 0x00000001.
Compliant Solution (NULL Error)
To rectify this problem, ensure that NULL is cast to an appropriate type when passing it to a variadic function.
Code Block | ||
---|---|---|
| ||
printf("%p %d\n", (void*)NULL, 1);
|
Risk Assessment
Inconsistent typing in variadic functions can result in abnormal program termination or unintended information disclosure.
...