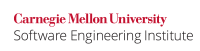
Copying data to a buffer that is not large enough to hold that data results in a buffer overflow. While Buffer overflows are not limited to null-terminated byte strings (NTBS), buffer overflows but they often occur when manipulating NTBS data. To prevent such errors, either limit copies through truncation or, preferably, ensure that the destination is of sufficient size to hold the character data to be copied and the null-termination character. (See STR03-C. Do not inadvertently truncate a null-terminated byte string.)
...
Command-line arguments are passed to main()
as pointers to null-terminated byte strings in the array members argv[0]
through argv[argc-1]
. If the value of argc
is greater than 0, the string pointed to by argv[0]
is, by convention, the program name. If the value of argc
is greater than 1, the strings referenced by argv[1]
through argv[argc-1]
are the actual program arguments.
Vulnerabilities can occur when inadequate space is allocated to copy a command-line argument or other program input. In this noncompliant code example, the contents of argv[0]
can be manipulated by an attacker to cause a buffer overflow:
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
/* Be prepared for argv[0] to be null. */
const char *const name = (argc && argv[0]) ? argv[0] : "";
char *prog_name = (char *)malloc(strlen(name) + 1);
if (prog_name != NULL) {
strcpy(prog_name, name);
} else {
/* Handle error */
}
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
#define __STDC_WANT_LIB_EXT1__ 1
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
/* Be prepared for argv[0] to be null. */
const char *const name = (argc && argv[0]) ? argv[0] : "";
char *prog_name;
size_t prog_size;
prog_size = strlen(name) + 1;
prog_name = (char *)malloc(prog_size);
if (prog_name != NULL) {
if (strcpy_s(prog_name, prog_size, name)) {
/* Handle error */
}
} else {
/* Handle error */
}
return 0;
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
int main(int argc, char *argv[]) {
/* Be prepared for argv[0] to be null. */
const char *const prog_name = (argc && argv[0]) ? argv[0] : "";
size_t prog_size;
return 0;
}
|
...
The getenv()
function searches an environment list, provided by the host environment, for a string that matches the string pointed to by name
. The set of environment names and the method for altering the environment list are implementation-defined. Environment variables can be arbitrarily large, and copying them into fixed-length arrays without first determining the size and allocating adequate storage can result in a buffer overflow.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h>
#include <string.h>
void func(void) {
char buff[256];
char *editor = getenv("EDITOR");
if (editor == NULL) {
/* EDITOR environment variable not set. */
} else {
strcpy(buff, editor);
}
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h>
#include <string.h>
void func(void) {
char *buff;
char *editor = getenv("EDITOR");
if (editor == NULL) {
/* EDITOR environment variable not set. */
} else {
size_t len = strlen(editor) + 1;
buff = (char *)malloc(len);
if (buff == NULL) {
/* Handle error */
}
memcpy(buff, editor, len);
}
}
|
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
|
| Can detect violations of the rule. However, it is unable to handle cases involving | |||||||
Coverity | 6.5 | STRING_OVERFLOW STRING_SIZE | Fully Implemented Fully implemented | ||||||
5.0 |
|
| |||||||
|
|
| |||||||
|
|
| |||||||
|
|
|
...
CERT C Secure Coding Standard | STR03-C. Do not inadvertently truncate a null-terminated byte string STR07-C. Use the bounds-checking interfaces for remediation of existing string manipulation code |
CERT C++ Secure Coding Standard | STR31-CPP. Guarantee that storage for character arrays has sufficient space for character data and the null terminator |
ISO/IEC TR 24772:2013 | String Termination [CJM] Buffer Boundary Violation (Buffer Overflow) [HCB] Unchecked Array Copying [XYW] |
ISO/IEC TS 17961 (Draft) | Using a tainted value to write to an object using a formatted input or output function [taintformatio] |
MITRE CWE | CWE-119, Failure to constrain operations within the bounds of an allocated memory buffer CWE-120, Buffer copy without checking size of input ("classic buffer overflow") CWE-193, Off-by-one error |
...