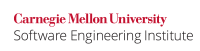
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> /* For malloc() and size_t */
struct flexArrayStruct {
int num;
int data[1];
};
/* ... */
size_t array_size;
size_t i;
void func(void) {
/* Initialize array_size */
/* Space is allocated for the struct. */
struct flexArrayStruct *structP
= (struct flexArrayStruct *)
malloc(sizeof(struct flexArrayStruct)
+ sizeof(int) * (array_size - 1));
if (structP == NULL) {
/* Handle malloc failure */
}
structP->num = 0;
/* Access data[] as if it had been allocated
* as data[array_size] */
for (i = 0; i < array_size; i++) {
structP->data[i] = 1;
}
} |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> /* For malloc() and size_t */
struct flexArrayStruct{
int num;
int data[];
};
/* ... */
size_t array_size;
size_t i;
void func(void) {
/* Initialize array_size */
/* Space is allocated for the struct. */
struct flexArrayStruct *structP = (struct flexArrayStruct *)
malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size);
if (structP == NULL) {
/* Handle malloc failure */
}
structP->num = 0;
/* Access data[] as if it had been allocated
* as data[array_size]
*/
for (i = 0; i < array_size; i++) {
structP->data[i] = 1;
}
} |
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DCL38-C | lowLow | unlikelyUnlikely | lowLow | P3 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
| Can detect some violations of this rule. In particular, it warns if the last element of a |
...