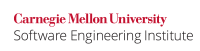
Flexible array members are a special type of array where the last element of a struct structure with more than one named member has an incomplete array type; that is, the size of the array is not specified explicitly within the structstructure.
If a dynamically sized structure is needed, flexible array members should be used.
...
In the following non-compliant code, an array of size 1 is declared, but when the struct structure itself is instantiated, the size computed for malloc()
is modified to take into account the full size of the dynamic array.
Code Block | ||
---|---|---|
| ||
struct flexArrayStruct { int num; int data[1]; }; /* ... */ /* Space is allocated for the struct */ struct flexArrayStruct *structP = malloc(sizeof(struct flexArrayStruct) + sizeof(int) * (ARRAY_SIZE - 1)); if (!structP) { /* handle malloc failure */ } structP->num = SOME_NUMBER; /* Access data[] as if it had been allocated as data[ARRAY_SIZE] */ for (i = 0; i < ARRAY_SIZE; i++) { structP->data[i] = i; } |
Wiki Markup |
---|
However, in thethis abovenon-compliant code example, the only member that is guaranteed to be valid, by strict C99 definition, is {{structP->data\[0\]}}. ThusConsequently, for all {{i > 0}}, the results of the assignment are undefined. |
...
Code Block | ||
---|---|---|
| ||
struct flexArrayStruct{
int num;
int data[];
};
/* ... */
/* Space is allocated for the struct */
struct flexArrayStruct *structP = malloc(sizeof(struct flexArrayStruct) + sizeof(int) * ARRAY_SIZE);
if (!structP) {
/* handle malloc failure */
}
structP->num = SOME_NUMBER;
/* Access data[] as if it had been allocated as data[ARRAY_SIZE] */
for (i = 0; i < ARRAY_SIZE; i++) {
structP->data[i] = i;
}
|
Wiki Markup |
---|
TheThis priorcompliant methodsolution allows the structstructure to be treated as if it had declared the member {{data\[\]}} to be {{data\[ARRAY_SIZE\]}} in a waymanner that conforms to the C99 standard. |
However, some restrictions do apply. :
- The incomplete array type must be the last element within the
...
- structure.
- You
...
- cannot have an array of
...
- structure if the
...
- structure contains flexible array members
...
- .
- Structures that contain a flexible array member cannot be used as a member in the middle of another
...
- structure.
Risk Assessment
Although the non-compliant method approach results in undefined behavior, it will does work under most architectures.
...