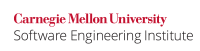
...
This code example is noncompliant because the character sequence c_str
will not be null-terminated when passed as an argument to printf()
. See (see STR11-C. Do not specify the bound of a character array initialized with a string literal.):
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> void func(void) { char c_str[3] = "abc"; printf("%s\n", c_str); } |
...
In this compliant solution, cur_msg
will always be null-terminated when passed to wcslen()
.:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> #include <wchar.h> wchar_t *cur_msg = NULL; size_t cur_msg_size = 1024; size_t cur_msg_len = 0; void lessen_memory_usage(void) { wchar_t *temp; size_t temp_size; /* ... */ if (cur_msg != NULL) { temp_size = cur_msg_size / 2 + 1; temp = realloc(cur_msg, temp_size * sizeof(wchar_t)); // temp & cur_msg might not be null-terminated if (temp == NULL) { /* Handle error */ } cur_msg = temp; // cur_msg now properly null-terminated cur_msg[temp_size - 1] = L'\0'; cur_msg_size = temp_size; cur_msg_len = wcslen(cur_msg); } } |
...