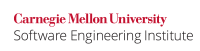
The C99 standard \[ [ISO/IEC 9899:1999|AA. Bibliography#ISO/IEC 9899-1999] \] introduces flexible array members into the language. While flexible array members are a useful addition, they need to be understood and used with care. Wiki Markup
The following is an example of a structure that contains a flexible array member:
...
Code Block | ||||
---|---|---|---|---|
| ||||
struct flexArrayStruct flexStruct; size_t array_size; size_t i; /* Initialize array_size */ /* Initialize structure */ flexStruct.num = 0; for (i = 0; i < array_size; i++) { flexStruct.data[i] = 0; } |
The problem with this code is that the {{ Wiki Markup flexArrayStruct
}} does not actually reserve space for the integer array data; it can't because the size hasn't been specified. Consequently, while initializing the {{num
}} member to zero is allowed, attempting to write even one value into data (that is, {{data
\[0
\]
}}) is likely to overwrite memory outside of the object's bounds.
Compliant Solution (Storage Allocation)
...
Code Block | ||||
---|---|---|---|---|
| ||||
struct flexArrayStruct *flexStruct; size_t array_size; size_t i; /* Initialize array_size */ /* Dynamically allocate memory for the structure */ flexStruct = (struct flexArrayStruct *)malloc( sizeof(struct flexArrayStruct) + sizeof(int) * array_size ); if (flexStruct == NULL) { /* Handle malloc failure */ } /* Initialize structure */ flexStruct->num = 0; for (i = 0; i < array_size; i++) { flexStruct->data[i] = 0; } |
...
The {{data
\[
\]
}} member of {{flexStruct
}} can now be accessed as described in C99, Section 6.7.2.1, paragraph 16.
Noncompliant Code Example (Copying)
...
Bibliography
\[[JTC1/SC22/WG14 N791|http://www.open-std.org/jtc1/sc22/wg14/www/docs/n791.htm]\] Wiki Markup
...
MEM32-C. Detect and handle memory allocation errors 08. Memory Management (MEM)