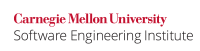
...
Furthermore, the definition of programmer-defined types may change. This creates a problem using these types with formatted output functions ( such as printf())
and formatted input functions ( such as scanf())
(see FIO00-C. Take care when creating format strings).
...
Code Block |
---|
mytypedef_t x; uintmax_t temp; /* ... */ temp = x; /* always safe secure*/ /* ... change the value of temp ... */ if (temp <= MYTYPEDEF_MAX) { x = temp; } |
Formatted I/O functions can be used to input and output greatest-width integer typed values. The j
length modifier in a format string indicates that the following d
, i
, o
, u
, x
, X
, or n
conversion specifier will apply to an argument with type intmax_t
or uintmax_t
. C99 also specifies the z
length modifier for use with arguments of type size_t
, and the t
length modifier for arguments of type ptrdiff_t
.
...
Code Block | ||
---|---|---|
| ||
#include <stdio.h> /* ... */ mytypedef_t x; /* ... */ printf("%llu", (unsigned long long) x); |
...
Code Block | ||
---|---|---|
| ||
#include <stdio.h> #include <inttypes.h> /* ... */ mytypedef_t x; /* ... */ printf("%ju", (uintmax_t) x); |
...
The following noncompliant code example can reads an unsigned long long
value from standard input and stores the result in x
, which is of a programmer-defined integer type.
Code Block | ||
---|---|---|
| ||
#include <stdio.h>
/* ... */
mytypedef_t x;
/* ... */
if (scanf("%llu", &x) != 1) {
/* handle error */
}
|
...
Code Block | ||
---|---|---|
| ||
#include <stdio.h> #include <inttypes.h> /* ... */ mytypedef_t x; uintmax_t temp; /* ... */ if (scanf("%ju", &temp) != 1) { /* handle error */ } if (temp > MYTYPEDEF_MAX) { /* handle error */ } else { x = temp; |
Risk Assessment
Failure to use an appropriate conversion specifier when inputting or outputting programmer-defined integer types can result in buffer overflow and lost or misinterpreted data.
...