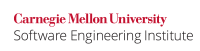
...
Code Block |
---|
errno_t strcpy_s( char * restrict s1, rsize_t s1max, char const * restrict s2 ); |
...
When a runtime-constraint violation is detected, the destination string is set to the NULL null string (as long as it is not a null pointer and the maximum length of the destination buffer is greater than zero and not greater than RSIZE_MAX
) and the function returns a nonzero value. In the following example, the strcpy_s()
function is used to copy src1
to dst1
.
...
Wiki Markup |
---|
However, the call to copy {{src2}} to {{dst2}} fails because there is insufficient space available to copy the entire string, which consists of eight characters, to the destination buffer. As a result, {{r2}} is assigned a nonzero value and {{dst2\[0\]}} is set to the null character. |
Users of the ISO/IEC TR 24731-1 functions are less likely to introduce a security flaw because the size of the destination buffer and the maximum number of characters to append must be specified. ISO/IEC TR 24731 Part II (24731-2, in progress) will offer another approach, supplying functions that allocate enough memory for their results. ISO/IEC TR 24731 functions also ensure NULL null termination of the destination string.
ISO/IEC TR 24731-1 functions are still capable of overflowing a buffer if the maximum length of the destination buffer and number of characters to copy are incorrectly specified. ISO/IEC TR 24731-2 functions may make it more difficult to keep track of memory that must be freed, leading to memory leaks. As a result, the ISO/IEC TR 24731 functions are not especially secure but may be useful in preventive maintenance to reduce the likelihood of vulnerabilities in an existing legacy code base.
...
Code Block | ||
---|---|---|
| ||
void complain(char const *msg) {
errno_t err;
static char const prefix[] = "Error: ";
static char const suffix[] = "\n";
char buf[BUFSIZ];
/* Ensure that more than one character
* is available for msg. */
static_assert(sizeof(buf) > sizeof(prefix) + sizeof(suffix),
"Buffer for complain() is too small");
strcpy(buf, prefix);
err = strcat_s(buf, sizeof(buf), msg);
if (err != 0) {
/* handle error */
}
err = strcat_s(buf, sizeof(buf), suffix);
if (err != 0) {
/* handle error */
}
fputs(buf, stderr);
}
|
...