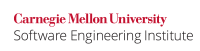
...
Function | Domain | Range |
---|---|---|
| -1 <= x && x <= 1 | no |
| x != 0 || y != 0 | no |
| x >= 1 | no |
| -1 < x && x < 1 | no |
none | yes | |
| none | yes |
| none | yes |
| x > 0 | no |
| x > -1 | no |
| x != 0 | yes |
| none | yes |
| none | yes |
x > 0 || (x == 0 && y > 0) || | yes | |
x >= 0 | no | |
| none | yes |
| ( x =!= 0 ) ||&& | yes |
| no none | yes |
| y != 0 | no |
| none | yes |
| none | yes |
| none | yes |
...
Range errors can usually not be prevented, so the most reliable way to handle range errors is to detect when they have occurred and act accordingly. The following approach uses C99 standard functions for floating point errors when the C99 macro math_errhandling
is defined and indicates that they should be used, otherwise it examines errno
.
Code Block |
---|
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; /* call the function */ #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* handle range error */ } #endif |
...
However, this code may produce a domain error if x
is negative and y
is not an integer, or if x
is zero and y
is zero. A domain error or range error may occur if x
is zero and y
is negative, and a range error may occur if the result cannot be represented as a double
.
...
Compliant Solution
Since the pow()
function can produce both domain errors and range errors, we must first check that x
and y
lie within the proper domain. We must also detect if a range error occurs, and act accordingly.
...