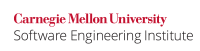
Integer conversions, both implicit and explicit (using a cast), must be guaranteed not to result in lost or misinterpreted data. This rule is particularly true for integer values that originate from untrusted sources and are used in any of the following ways:
...
The only integer type conversions that are guaranteed to be safe for all data values and all possible conforming implementations are conversions of an integral value to a wider type of the same signedness. The C Standard, subclause 6.3.1.3 [ISO/IEC 9899:2011], says,
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h> void func(void) { signed int si = INT_MIN; unsigned int ui; if (si < 0) { /* Handle error */ } else { ui = (unsigned int)si; /* Cast eliminates warning */ } /* ... */ } |
Subclause 6.2.5, paragraph 9, of the C Standard [ISO/IEC 9899:2011] provides the necessary guarantees to ensure this solution works on an a conforming implementation:
The range of nonnegative values of a signed integer type is a subrange of the corresponding unsigned integer type, and the representation of the same value in each type is the same.
...
For historical reasons, certain C Standard functions accept an argument of type int
and convert it to either unsigned char
or plain char
. This conversion can result in a unexpected behavior if the value cannot be represented in the smaller type. This noncompliant solution unexpectedly clears the array.:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <string.h> #include <stddef.h> #include <limits.h> int *init_memory(int *array, size_t n) { return memset(array, 4096, n); } |
...
INT31-EX1: The C Standard defines minimum ranges for standard integer types. For example, the minimum range for an object of type unsigned short int
is 0 to 65,535, whereas the minimum range for int
is −32,767 to +32,767. Consequently, it is not always possible to represent all possible values of an unsigned short int
as an int
. However, on the IA-32 architecture, for example, the actual integer range is from −2,147,483,648 to +2,147,483,647, meaning that it is quite possible to represent all the values of an unsigned short int
as an int
for this architecture. As a result, it is not necessary to provide a test for this conversion on IA-32. It is not possible to make assumptions about conversions without knowing the precision of the underlying types. If these tests are not provided, assumptions concerning precision must be clearly documented, as the resulting code cannot be safely ported to a system where these assumptions are invalid. A good way to document these assumptions is to use static assertions (see DCL03-C. Use a static assertion to test the value of a constant expression).
INT31-EX2: Conversion from any integer type with a value between SCHAR_MIN
and UCHAR_MAX
to a character type is permitted provided the value represents a character and not an integer.
Conversions to unsigned character types are well-defined by C to have modular behavior. A character's value is not misinterpreted by the loss of sign or conversion to a negative number. For example, the Euro symbol €
is sometimes represented by bit pattern 0x80
which can have the numerical value 128 or -127 −127 depending on the signedness of the type.
Conversions to signed character types are more problematic. The C Standard, subclause 6.3.1.3, paragraph 3 [ISO/IEC 9899:2011], says, regarding conversions:
...
NOTE: Two's complement is shorthand for "radix complement in radix 2." Ones' complement is shorthand for "diminished radix complement in radix 2."
Consequently, the standard allows for this code to trap:
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| ALLOC.SIZE.TRUNC | Truncation of Allocation Sizeallocation size | ||||||
Can detect violations of this rule. However, false warnings may be raised if | |||||||||
| NEGATIVE_RETURNS
| Can find array accesses, loop bounds, and other expressions that may contain dangerous implied integer conversions that would result in unexpected behavior Can find instances where a negativity check occurs after the negative value has been used for something else Can find instances where an integer expression is implicitly converted to a narrower integer type, where the signedness of an integer value is implicitly converted, or where the type of a complex expression is implicitly converted | |||||||
Cppcheck |
| memsetValueOutOfRange | The 2nd argument second argument to memset() cannot be represented as unsigned char . | ||||||
5.0 | Can detect violations of this rule with CERT C Rule Pack | ||||||||
| PRECISION.LOSS | ||||||||
| 93 S | Fully implemented | |||||||
PRQA QA-C |
| 2850, 2851, 2852, 2853, | Partially implemented |
...
CVE-2009-1376 results from a violation of this rule. In version 2.5.5 of Pidgin, a size_t
offset is set to the value of a 64-bit unsigned integer, which can lead to truncation [xorl 2009] on platforms where a size_t
is implemented as a 32-bit unsigned integer. An attacker can execute arbitrary code by carefully choosing this value and causing a buffer overflow.
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...