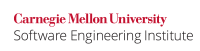
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stddef.h> size_t getlen(const int *input, size_t maxlen, int delim) { for (size_t i = 0; i < maxlen; ++i) { if (input[i] == delim) { return i; } } } void func(int userdata) { size_t i; int data[] = { 1, 1, 1 }; i = getlen(data, sizeof(data), 0); data[i] = userdata; } |
Implementation Details
When the following is compiled with -Wall
on most versions of the GCC compiler, the following warning is generated:
Code Block |
---|
example.c: In function 'getlen':
example.c:12: warning: control reaches end of non-void function
|
When the following program is compiled and run with GCC 4.4.3 on Linux, the value of i
is 5, as if the getlen()
function returned 5, causing an out-of-bounds write to the data
array:
The following program violates this rule:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> size_t getlen(const int *input, size_t maxlen, int delim) { for (size_t i = 0; i < maxlen; ++i) { if (input[i] == delim) { return i; } } } int main(int argc, char **argv) { size_t i; int data[] = { 1, 1, 1 }; i = getlen(data, sizeof(data), 0); printf("Returned: %zu\n", i); data[i] = 0; return 0; } |
When this program is compiled with -Wall
on most versions of the GCC compiler, the following warning is generated:
Code Block |
---|
example.c: In function 'getlen':
example.c:12: warning: control reaches end of non-void function
|
When run with GCC 4.4.3 on Linux, the value of i
is 5, as if the getlen()
function returned 5, causing an out-of-bounds write to the data
array.
Compliant Solution
This compliant solution changes the interface of getlen()
to store the result in a user-provided pointer and return an error code to indicate any error conditions. The best method for handling this type of error is specific to the application and the type of error (see ERR00-C. Adopt and implement a consistent and comprehensive error-handling policy for more on error handling).
...