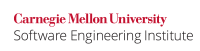
...
Code Block | ||
---|---|---|
| ||
struct buffer { size_t size; char buffer[50]; }; ... void func(struct buffer *buf) { /* assuming sizeof(size_t) is 4, sizeof(size_t)+sizeof(char)*50 equals 54 */ struct buffer *buf_cpy = malloc(sizeof(size_t)+(sizeof(char)*50)); if (buf_cpy == NULL) { /* Handle malloc() error */ } ... /* with padding, sizeof(struct buffer) may be greater than 54, causing a small amount of data to be written outside the bounds of the memory allocated */ memcpy(buf_cpy, buf, sizeof(struct buffer)); } |
Risk Assessment
Failure to correctly determine the size of a structure can lead to subtle logic errors and buffer overflows.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
STR33-C | 3 (medium) | 3 (probable) | 2 (medium) | P18 | L1 |
Compliant Solution
Accounting for structure padding prevents these types of errors.
Code Block | ||
---|---|---|
| ||
struct buffer { size_t size; char buffer[50]; }; ... void func(struct buffer *buf) { struct buffer *buf_cpy = malloc((sizeof(struct buffer)); if (buf_cpy == NULL) { /* Handle malloc() error */ } ... memcpy(buf_cpy, buf, sizeof(struct buffer)); } |
Risk Assessment
Failure to correctly determine the size of a structure can lead to subtle logic errors and buffer overflows.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP03-A |
|
|
|
|
|
References
Wiki Markup |
---|
\[[Dowd 06|AA. C References#Dowd 06]\] Chapter 6, "C Language Issues" (Structure Padding 284-287) \[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\] Section 6.7.2.1, "Structure and union specifiers" |
...