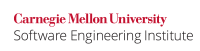
...
Code Block | ||
---|---|---|
| ||
void foo(int *x) { if (x != NULL) { *x = 3; /* visible outside function */ } /* ... */ } |
...
Compliant Solution
In this noncompliant code example, the function parameter is const
-qualified. Any attempt to modify the pointed-to value is diagnosed by the compiler.
Code Block | ||
---|---|---|
| ||
void foo(const int * x) { if (x != NULL) { *x = 3; /* generates compiler error */ } /* ... */ } |
Compliant Solution
Consequently the compiler will refuse to compile this function, forcing the programmer to solve the const violation.
Compliant Solution
This compliant solution addresses the const violation by not modifying the constant argumentIf a function does not modify the pointed-to value, it should declare this value as const
. This improves code readability and consistency.
Code Block | ||
---|---|---|
| ||
void foo(const int * x) { if (x != NULL) { printf("Value is %d\n", *x); } /* ... */ } |
Noncompliant Code Example
This noncompliant code example defines a fictional version of the standard strcat()
function called strcat_nc()
. This function differs from strcat()
in that the second argument is not const
-qualified.
...
In the final strcat_nc()
call, the compiler generates a warning about attempting to cast away const
on str4
. This is a valid warning.
Compliant Solution
This compliant solution uses the prototype for the strcat()
from C90. Although the restrict
type qualifier did not exist in C90, const
did. In general, function parameters should be declared in a manner consistent with the semantics of the function. In the case of strcat()
, the initial argument can be changed by the function while the second argument cannot.
...