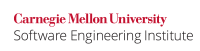
Some environments implomentations provide a nonportable environment pointers pointer that are is valid when main()
is called but may be invalided invalidated by operations that modify the environment.
Subclause The C Standard, J.5.1 of the C Standard [ISO/IEC 9899:2011], states:
In a hosted environment, the main function receives a third argument,
char *envp[]
, that points to a null-terminated array of pointers tochar
, each of which points to a string that provides information about the environment for this execution of the program.
Consequently, under a hosted environment supporting this common extension, it is possible to access the environment through a modified form of main()
:
Code Block |
---|
main(int argc, char *argv[], char *envp[]){ /* ... */ }
|
However, modifying the environment by any means may cause the environment memory to be reallocated, with the result that envp
now references an incorrect location. For example, when compiled with GCC 4.8.1 and run on a 32-bit Intel GNU/Linux machine, the following code,
...
It is evident from these results that the environment has been relocated as a result of the call to setenv()
. The external variable environ
is updated to refer to the current environment; the envp
parameter is not.
An environment pointer may also become invalidated by subsequent calls to getenv()
.See (see ENV34-C. Do not store pointers returned by certain functions for more information).
Noncompliant Code Example (POSIX)
After a call to the POSIX setenv()
function or to another function that modifies the environment, the envp
pointer may no longer reference the current environment. Standard for Information Technology—Portable The Portable Operating System Interface (POSIX®POSIX®), Base Specifications, Issue 7 [IEEE Std 1003.1:2013], states:
Unanticipated results may occur if
setenv()
changes the external variableenviron
. In particular, if the optionalenvp
argument tomain()
is present, it is not changed, and thus may point to an obsolete copy of the environment (as may any other copy ofenviron
).
This noncompliant code example accesses the envp
pointer after calling setenv()
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> int main(int argc, const char *argv[], const char *envp[]) { if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle error */ } if (envp != NULL) { for (size_t i = 0; envp[i] != NULL; ++i) { if (puts(envp[i]) == EOF) { /* Handle error */ }; } } return 0; } |
Because envp
may no longer point to the current environment, this program has undefined behavior.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> extern char **environ; int main(int argc, const char *argv[]void) { if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle error */ } if (environ != NULL) { for (size_t i = 0; environ[i] != NULL; ++i) { if (puts(environ[i]) == EOF) { ; /* Handle error */ } } } return 0; } |
Noncompliant Code Example (Windows)
...
This noncompliant code example accesses the envp
pointer after calling _putenvsputenv_s()
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> int main(int argc, const char *argv[], const char *envp[]) { if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle error */ } if (envp != NULL) { for (size_t i = 0; envp[i] != NULL; ++i) { if (puts(envp[i]) == EOF) { /* Handle error */ }; } } return 0; } |
Because envp
no longer points to the current environment, this program fails to print the value of MY_NEW_VAR
has undefined behavior.
Compliant Solution (Windows)
Use This compliant solution uses the _environ
variable in place of envp
when defined:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> _CRTIMP extern char **_environ; int main(int argc, const char *argv[]) { size_t i; if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle error */ } if (_environ != NULL) { for (size_t i = 0; _environ[i] != NULL; ++i) { if (puts(_environ[i]) == EOF) { /* Handle error */ ; } } } return 0; } |
Compliant Solution
If you have a great deal of unsafe envp
code, you can save time in your remediation by replacingThis compliant solution can reduce remediation time when a large amount of noncompliant envp
code exists. It replaces
Code Block |
---|
int main(int argc, char *argv[], char *envp[]) { /* ... */ } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#if defined (_POSIX_) || defined (__USE_POSIX)
extern char **environ;
#define envp environ
#elif defined(_WIN32)
_CRTIMP extern char **_environ;
#define envp _environ
#endif
int main(int argc, char *argv[]) {
/* ... */
}
|
This compliant solution may need to be extended to support other implementations that support forms of the external variable environ
.
Risk Assessment
Using the envp
environment pointer after the environment has been modified can result in undefined behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ENV31-C | Low | Probable | Medium | P4 | L3 |
...
Search for vulnerabilities resulting, from the violation of this _putenv_s
, rule on the CERT website.
Related Guidelines
...
[IEEE Std 1003.1:2013] | XSH, System Interfaces, setenv |
[ISO/IEC 9899:2011] | Subclause J.5.1, "Environment Arguments" |
[MSDN] | , ,
getenv , _wgetenv ,_putenv_s , _wputenv_s |
...