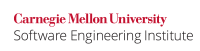
...
See FLP03-C. Detect and handle floating-point errors for more details on how to detect floating-point errors.
...
Denormalized Numbers
Certain functions may produce range errors specifically when applied with a denormal argumentdenormalized number. These functions are: asin()
, asinh()
, atan()
, atanh()
, and erf()
. When evaluated with a denormal argumentdenormalized number, these functions can produce an inexact, denormal valuedenormalized value, which is an underflow error. Subclause 7.12.1, paragraph 6, of the C Standard [ISO/IEC 9899:2011] defines the following behavior for floating-point underflow:
...
Function | Arguments |
---|---|
fmod() | ((min+denorm),min) |
remainder() | ((min+denorm),min) |
remquo() | ((min+denorm),min,quo) |
If denormal results denormalized results are supported, the returned value is exact and there will not be a range error. When dealing with fmod()
, subclause F.10.7.1, paragraph 2, of the C Standard [ISO/IEC 9899:2011] states:
...
Subclause F.10.7.2, paragraph 2, and subclause F.10.7.3, paragraph 2 of the C Standard [ISO/IEC 9899:2011] cover remainder()
and remquo()
for when denormal results denormalized results are supported.
Noncompliant Code Example (sqrt()
)
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <errno.h> #include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #pragma STDC FENV_ACCESS ON #endif void func(double x, double y) { #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; double result; if (((x == 0.0f) && islessequal(y, 0.0)) || isless(x, 0.0)) { /* Handle domain or pole error */ } result = pow(x, y); #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* Handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* Handle range error */ } #endif } |
Noncompliant Code Example (asin()
,
...
Denormalized Number)
This noncompliant code example determines the arcsin of x:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <math.h> void func(float x) { float result = asin(x); /* ... */ } |
Compliant Soluction (asin()
,
...
Denormalized Number)
Because this function has no domain errors but may have range errors, the programmer must detect a range error and act accordingly:
...