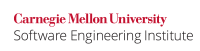
Strings In C, a string must contain a null-termination character by definition. This null-termination character exists at or before the address of the last element of the array containing the string. Character arrays must be null-terminated before they can may be safely passed as arguments to standard string-handling functions, such as strcpy()
or strlen()
. These functions, as well as other string-handling functions defined by the C Standard, depend on the existence of the string's null-termination character to determine the length of the string. SimilarlyLikewise, strings character sequences must be null-terminated before iterating on a character array the sequence where the termination condition of the loop depends on the existence of a null-termination character within the memory allocated for the stringsequence, as in the following example:
Code Block | ||
---|---|---|
| ||
void func(void) { char ntbs[16]; for (size_t i = 0; i < sizeof(ntbs); ++i) { if (ntbs[i] == '\0') { break; } } |
Failure to properly terminate strings Passing a non-null-terminated character array to a function that expects a string can result in buffer overflows and other undefined behavior.
Noncompliant Code Example (strncpy()
)
The While the strncpy()
function takes a string as input, it does not guarantee that the resulting value is still null-terminated. If In the following noncompliant code example, if no null character is contained in the first n
characters of the source
array, the result will not be null-terminated. Passing a non-null-terminated character sequence to strlen()
results in undefined behavior, as shown by this noncompliant code example:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <string.h> enum { NTBS_SIZE = 32 }; size_t func(const char *source) { char ntbs[NTBS_SIZE]; ntbs[sizeof(ntbs) - 1] = '\0'; strncpy(ntbs, source, sizeof(ntbs)); return strlen(ntbs); } |
...
If the intent is to copy without truncation, this example copies the data and guarantees that the resulting string array is null-terminated. If the string cannot be copied, it is handled as an error condition.
...
Because realloc()
does not guarantee that the string character sequence is properly null-terminated, and the function subsequently passes cur_msg
to a library function (fputs()
) that expects null-termination, the result is undefined behavior.
...