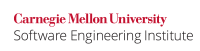
C has very weak typing. It lets you Weak typing in C allows type-cast casting memory to different types, allowing you to apply operations . Since the internal representation of most types is system-dependent, applying operations intended for data of one type to data of a different type . However, the internal representation of most types are system-dependent. Applying operations on improper types will likely yield non-portable code and produce unexpected results.
...
The following non-compliant code demonstrates the perils of operating on data of improper incompatible types. It tries An attempt is made to increment an int integer type cast as to a floatfloating point type, and a float type floating point cast as an int, and displays the resultsto an integer type.
Code Block | ||
---|---|---|
| ||
#include <assert.h> #include <stdio.h> int main(void) { float f = 0.0; int i = 0; float *fp; int *ip; assert(sizeof(int) == sizeof(float)); ip = (int*) &f; fp = (float*) &i; printf("int is %d, float is %f\n", i, f); (*ip)++; (*fp)++; printf("int is %d, float is %f\n", i, f); return 0; } |
The expected result is for both values to display as 1
, however, Rather than the int and float both having the value 1, on a 64-bit Linux machine, this program produces:
...
In this compliant solution, the pointers are assigned to the variables of the proper compatible data types.
Code Block | ||
---|---|---|
| ||
#include <stdio.h> int main(void) { float f = 0.0; int i = 0; float *fp; int *ip; ip = &i; fp = &f; printf("int is %d, float is %f\n", i, f); (*ip)++; (*fp)++; printf("int is %d, float is %f\n", i, f); return 0; } |
On This program, on the same platform, this solution produces the expected output of:
Code Block |
---|
int is 0, float is 0.000000 int is 1, float is 1.000000 |
...
Bit-Fields
The internal representation representations of bit-field structs structures have several properties (such as internal padding) that are implementation-defined. For instance, they may contain internal padding. BitAdditionally, bit-field structures have several additional implementation-defined constraints:
...
If each bit-field lives within its own byte, then m2
(or m1
, depending on alignment) is incremented by 1. If the bit-fields are indeed packed across 8-bit bytes, then m2
might be incremented by 4.This code also violates ARR37-C. Do not add or subtract an integer to a pointer to a non-array object.
Compliant Solution (Bit-Field Overlap)
This compliant solution is also explicit in which fields it modifies.
...