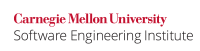
...
This noncompliant code attempts to allocated a flexible array member with a one element array as the final member. When the structure is instantiated, the size computed for malloc()
is modified to account for the actual size of the dynamic array.
Code Block | ||||
---|---|---|---|---|
| ||||
struct flexArrayStruct { int num; int data[1]; }; /* ... */ size_t array_size; size_t i; /* initialize array_size */ /* space is allocated for the struct */ struct flexArrayStruct *structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * (array_size - 1)); if (structP == NULL) { /* Handle malloc failure */ } structP->num = 0; /* access data[] as if it had been allocated * as data[array_size] */ for (i = 0; i < array_size; i++) { structP->data[i] = 1; } |
...
This compliant solution uses the flexible array member to achieve a dynamically sized structure.
Code Block | ||||
---|---|---|---|---|
| ||||
struct flexArrayStruct{ int num; int data[]; }; /* ... */ size_t array_size; size_t i; /* Initialize array_size */ /* Space is allocated for the struct */ struct flexArrayStruct *structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (structP == NULL) { /* Handle malloc failure */ } structP->num = 0; /* Access data[] as if it had been allocated * as data[array_size] */ for (i = 0; i < array_size; i++) { structP->data[i] = 1; } |
...