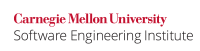
...
Type range errors, including loss of data (truncation) and loss of sign (sign errors), can occur when converting from an unsigned type to a signed type. The following noncompliant code example results in a truncation error on most implementations.
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned long int ul = ULONG_MAX; signed char sc; sc = (signed char)ul; /* cast eliminates warning */ |
...
Validate ranges when converting from an unsigned type to a signed type. The following code, for example, can be used when converting from unsigned long int
to a signed char
.
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned long int ul = ULONG_MAX; signed char sc; if (ul <= SCHAR_MAX) { sc = (signed char)ul; /* use cast to eliminate warning */ } else { /* handle error condition */ } |
...
Type range errors, including loss of data (truncation) and loss of sign (sign errors), can occur when converting from a signed type to an unsigned type. The following code results in a loss of sign.
Code Block | ||||
---|---|---|---|---|
| ||||
signed int si = INT_MIN; unsigned int ui = (unsigned int)si; /* cast eliminates warning */ |
...
Validate ranges when converting from a signed type to an unsigned type. The following code, for example, can be used when converting from signed int
to unsigned int
.
Code Block | ||||
---|---|---|---|---|
| ||||
signed int si = INT_MIN; unsigned int ui; if (si < 0) { /* handle error condition */ } else { ui = (unsigned int)si; /* cast eliminates warning */ } |
...
A loss of data (truncation) can occur when converting from a signed type to a signed type with less precision. The following code can result in truncation.
Code Block | ||||
---|---|---|---|---|
| ||||
signed long int sl = LONG_MAX; signed char sc = (signed char)sl; /* cast eliminates warning */ |
...
Validate ranges when converting from a signed type to a signed type with less precision. The following code can be used, for example, to convert from a signed long int
to a signed char
.
Code Block | ||||
---|---|---|---|---|
| ||||
signed long int sl = LONG_MAX; signed char sc; if ( (sl < SCHAR_MIN) || (sl > SCHAR_MAX) ) { /* handle error condition */ } else { sc = (signed char)sl; /* use cast to eliminate warning */ } |
...
A loss of data (truncation) can occur when converting from an unsigned type to an unsigned type with less precision. The following code results in a truncation error on most implementations.
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned long int ul = ULONG_MAX; unsigned char uc = (unsigned char)ul; /* cast eliminates warning */ |
...
Validate ranges when converting from an unsigned type to an unsigned type with lesser precision. The following code can be used, for example, to convert from an unsigned long int
to an unsigned char
.
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned long int ul = ULONG_MAX; unsigned char uc; if (ul > UCHAR_MAX) ) { /* handle error condition */ } else { uc = (unsigned char)ul; /* use cast to eliminate warning */ } |
...