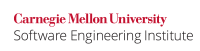
...
This noncompliant code example prints the value of x
as an unsigned long long
value, even though the value is of a programmer-defined integer type.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> mytypedef_t x; /* ... */ printf("%llu", (unsigned long long) x); |
...
This compliant solution guarantees that the correct value of x
is printed, regardless of its length, provided that mytypedef_t
is an unsigned type.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <inttypes.h> mytypedef_t x; /* ... */ printf("%ju", (uintmax_t) x); |
...
The following noncompliant code example reads an unsigned long long
value from standard input and stores the result in x
, which is of a programmer-defined integer type.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> mytypedef_t x; /* ... */ if (scanf("%llu", &x) != 1) { /* handle error */ } |
...
This compliant solution guarantees that a correct value in the range of mytypedef_t
is read, or an error condition is detected, assuming the value of MYTYPEDEF_MAX
is correct as the largest value representable by mytypedef_t
.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <inttypes.h> mytypedef_t x; uintmax_t temp; /* ... */ if (scanf("%ju", &temp) != 1) { /* handle error */ } if (temp > MYTYPEDEF_MAX) { /* handle error */ } else { x = temp; |
...