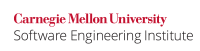
...
The following noncompliant code demonstrates the perils of operating on data of incompatible types. An attempt is made to increment an integer type cast to a floating point type and a floating point cast to an integer type.
Code Block | ||||
---|---|---|---|---|
| ||||
float f = 0.0; int i = 0; float *fp; int *ip; assert(sizeof(int) == sizeof(float)); ip = (int*) &f; fp = (float*) &i; printf("int is %d, float is %f\n", i, f); (*ip)++; (*fp)++; printf("int is %d, float is %f\n", i, f); |
...
In this compliant solution, the pointers are assigned to reference variables of compatible data types.
Code Block | ||||
---|---|---|---|---|
| ||||
float f = 0.0; int i = 0; float *fp; int *ip; ip = &i; fp = &f; printf("int is %d, float is %f\n", i, f); (*ip)++; (*fp)++; printf("int is %d, float is %f\n", i, f); |
...
The following code behaves differently depending on whether the implementation is left-to-right or right-to-left:
Code Block | ||||
---|---|---|---|---|
| ||||
struct bf { unsigned int m1 : 8; unsigned int m2 : 8; unsigned int m3 : 8; unsigned int m4 : 8; }; /* 32 bits total */ void function() { struct bf data; unsigned char *ptr; data.m1 = 0; data.m2 = 0; data.m3 = 0; data.m4 = 0; ptr = (unsigned char *)&data; (*ptr)++; /* can increment data.m1 or data.m4 */ } |
...
This compliant solution is explicit in which fields it modifies.
Code Block | ||||
---|---|---|---|---|
| ||||
struct bf { unsigned int m1 : 8; unsigned int m2 : 8; unsigned int m3 : 8; unsigned int m4 : 8; }; /* 32 bits total */ void function() { struct bf data; data.m1 = 0; data.m2 = 0; data.m3 = 0; data.m4 = 0; data.m1++; } |
...
In the following noncompliant code, assuming eight bits to a byte, if bit-fields of six and four bits are declared, is each bit-field contained within a byte, or are the bit-fields split across multiple bytes?
Code Block | ||||
---|---|---|---|---|
| ||||
struct bf { unsigned int m1 : 6; unsigned int m2 : 4; }; void function() { unsigned char *ptr; struct bf data; data.m1 = 0; data.m2 = 0; ptr = (unsigned char *)&data; ptr++; *ptr += 1; /* what does this increment? */ } |
...
This compliant solution is explicit in which fields it modifies.
Code Block | ||||
---|---|---|---|---|
| ||||
struct bf { unsigned int m1 : 6; unsigned int m2 : 4; }; void function() { struct bf data; data.m1 = 0; data.m2 = 0; data.m2 += 1; } |
...