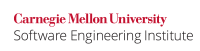
A signal handler should not re-assert its desire to handle its own signal. This is often done on non-persistent platforms, that is, when a signal handler receives a signal that is bound to a handler, they unbind the signal to default behavior before calling the handler.
A signal handler may only call signal()
to reset the signal, or change the current way signals are handled. if it does not need to be async-safe (in other words, all relevant signals are masked, and it may therefore not be interrupted.)
Non-Compliant Code Example
...
Code Block | ||
---|---|---|
| ||
void handler(int signum) { /* handling code */ } /* ... */ /* Equivalent to signal( signum, handler); but make signal persistent */ struct sigaction act; act.sa_handler = &handler; act.sa_flags = 0; if (sigemptyset( &act.sa_mask) != 0) { /* handle error */ } if (sigaction(signum, &act, NULL) != 0) { /* handle error */ } |
While the handler in this example does not call signal()
, it safely can, since relevant signals are masked and so the handler can not be interrupted.
In fact, POSIX recommends sigaction(2)
and deprecates signal(2)
. Unfortunately, sigaction(2)
is not C99-compliant, and is not supported on some platforms, including Windows.
...