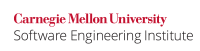
...
Code Block | ||
---|---|---|
| ||
signed int si1, si2, sum; if ( ((si1^si2) | (((si1^(~(si1^si2) & (1 << (sizeof(int)*CHAR_BIT-1))))+si2)^si2)) >= 0) { /* handle error condition */ } sum = si1 + si2; |
...
Code Block | ||
---|---|---|
| ||
signed int si1, si2, result; if (((si1^si2) & (((si1 ^ ((si1^si2) & (1 << (sizeof(int)*CHAR_BIT-1))))-si2)^si2)) < 0) { /* handle error condition */ } result = si1 - si2; |
...
Code Block | ||
---|---|---|
| ||
signed int si1, si2, result; static_assert( sizeof(long long) >= sizeof(int), "Unable to detect overflow after multiplication" ); signed long long tmp = (signed long long)si1 * (signed long long)si2; /* * If the product cannot be represented as a 32-bit integer, * handle as an error condition. */ if ( (tmp > INT_MAX) || (tmp < INT_MIN) ) { /* handle error condition */ } result = (int)tmp; |
...
Code Block | ||
---|---|---|
| ||
int si1, si2, sresult; if ( (si1 < 0) || (si2 < 0) || (si2 >= sizeof(int)*CHAR_BIT) || si1 > (INT_MAX >> si2) ) { /* handle error condition */ } else { sresult = si1 << si2; } |
...