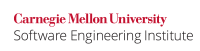
...
The following non-compliant code example fails to ensure that cur_msg
is properly null - terminated:
Code Block | ||
---|---|---|
| ||
char *cur_msg = NULL; size_t cur_msg_size = 1024; /* ... */ void lessen_memory_usage(void) { char *temp; size_t temp_size; /* ... */ if (cur_msg != NULL) { temp_size = cur_msg_size/2 + 1; temp = realloc(cur_msg, temp_size); if (temp == NULL) { /* Handle error condition */ } cur_msg = temp; cur_msg_size = temp_size; } } /* ... */ |
Because realloc()
does not guarantee that the string is properly null - terminated, any subsequent operation on cur_msg
that assumes a null-termination character may result in undefined behavior.
...
In this compliant solution, the lessen_memory_usage()
function ensures that the resulting string is always properly null - terminated.
Code Block | ||
---|---|---|
| ||
char *cur_msg = NULL; size_t cur_msg_size = 1024; /* ... */ void lessen_memory_usage(void) { char *temp; size_t temp_size; /* ... */ if (cur_msg != NULL) { temp_size = cur_msg_size/2 + 1; temp = realloc(cur_msg, temp_size); if (temp == NULL) { /* Handle error condition */ } cur_msg = temp; cur_msg_size = temp_size; cur_msg[cur_msg_size - 1] = '\0'; /* ensure string is null-terminated */ } } /* ... */ |
Risk Assessment
Failure to properly null - terminate strings can result in buffer overflows and the execution of arbitrary code with the permissions of the vulnerable process. Null-termination errors can also result in unintended information disclosure.
...