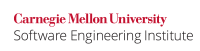
If ptr
was allocated with an alignment returned from aligned_alloc()
and if realloc()
reallocates memory with a different alignment then, the behavior is undefined.
...
This noncompliant code example aligns ptr
to a 4096-byte boundary, whereas the realloc()
function aligns the memory to a different alignment.
...
The resulting program has undefined behavior as because the alignment that realloc()
enforces is different from that of aligned_alloc()
function's alignment.
Implementation Details
When compiled with gcc version GCC Version 4.1.2 and run on the x86_64 -redhat-linux platform Red Hat Linux platform, the following code produces the following output:
CODE
Code Block |
---|
#include <stdlib.h> #include <stdio.h> int main(void) { size_t size = 16; size_t resize = 1024; size_t align = 1 << 12; int *ptr; int *ptr1; if (posix_memalign((void **)&ptr, align , size) != 0) { exit(EXIT_FAILURE); } printf("memory aligned to %d bytes\n", align); printf("ptr = %p\n\n", ptr); if ((ptr1 = realloc((int *)ptr, resize)) == NULL) { exit(EXIT_FAILURE); } puts("After realloc(): \n"); printf("ptr1 = %p\n", ptr1); free(ptr1); return 0; } |
produces the following output:OUTPUT
Code Block |
---|
memory aligned to 4096 bytes ptr = 0x1621b000 After realloc(): ptr1 = 0x1621a010 |
...
This compliant solution implements an aligned realloc()
function. It allocates resize
bytes of new memory of resize
bytes with an alignment equal to that of the same alignment as the old memory and copies old memory into it. It then frees then moves the old memory there, consequently freeing up the old memory.
Code Block | ||
---|---|---|
| ||
size_t resize = 1024; size_t alignment = 1 << 12; int *ptr; int *ptr1; if ((ptr = aligned_alloc(alignment, sizeof(int))) == NULL) { /* handle error */ } /* ... */ if ((ptr1 = aligned_alloc(alignment, resize)) == NULL) { /* handle error */ } if((memcpy(ptr1, ptr, sizeof(int)) == NULL) { /* handle error */ } free(ptr); |
...
Improper alignment can lead to accessing arbitrary memory locations and write into itbeing accessed and written to.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC36-C | high | probable | medium | P12 | L1 |
...