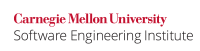
...
Operating System | Handling FP errors | ||
---|---|---|---|
Linux | C99 FP functions - These functions are declared in | AA. C References#Open Group 04]] | |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="4925df4936babb0b-8a17282e-404a4bd8-a1af8afa-e071438a41660965d42463b6"><ac:plain-text-body><![CDATA[ | Windows | Structured Exception Handling - user defined handler | AA. C References#MSDN]] |
...
Code Block | ||
---|---|---|
| ||
fpOper_noErrorChecking(void) {
/* ... */
double a = 1e-40, b, c = 0.1;
float x = 0, y;
/* inexact and underflows */
y = a;
/* divide by zero operation */
b = y / x;
/* inexact (loss of precision) */
c = sin(30) * a;
/* ... */
}
|
...
Code Block | ||
---|---|---|
| ||
#include <fenv.h>
fpOper_fenv(void) {
double a = 1e-40, b, c = 0.1;
float x = 0, y;
int fpeRaised;
/* ... */
feclearexcept(FE_ALL_EXCEPT);
/* Store a into y is inexact and underflows: */
y = a;
fpeRaised = fetestexcept(FE_ALL_EXCEPT);
/* fpeRaised has FE_INEXACT and FE_UNDERFLOW */
feclearexcept(FE_ALL_EXCEPT);
/* divide by zero operation */
b = y / x;
fpeRaised = fetestexcept(FE_ALL_EXCEPT);
/* fpeRaised has FE_DIVBYZERO */
feclearexcept(FE_ALL_EXCEPT);
c = sin(30) * a;
fpeRaised = fetestexcept(FE_ALL_EXCEPT);
/* fpeRaised has FE_INEXACT */
feclearexcept(FE_ALL_EXCEPT);
/* ... */
}
|
...
Code Block | ||
---|---|---|
| ||
fpOper_usingStatus(void) {
/* ... */
double a = 1e-40, b, c;
float x = 0, y;
unsigned int rv = _clearfp() ;
/* Store into y is inexact and underflows: */
y = a;
rv = _clearfp() ; /* rv has _SW_INEXACT and _SW_UNDERFLOW */
/* zero-divide */
b = y / x; rv = _clearfp() ; /* rv has _SW_ZERODIVIDE */
/* inexact */
c = sin(30) * a; rv = _clearfp() ; /* rv has _SW_INEXACT */
/* ... */
}
|
...
Code Block | ||
---|---|---|
| ||
fp_usingSEH(void) {
/* ... */
double a = 1e-40, b, c = 0.1;
float x = 0, y;
unsigned int rv ;
unmask_fp();
_try {
/* Store into y is inexact and underflows */
y = a;
/* divide by zero operation */
b = y / x;
/* inexact */
c = sin(30) * a;
}
_except (_fpieee_flt (GetExceptionCode(), GetExceptionInformation(), fpieee_handler)) {
printf ("fpieee_handler: EXCEPTION_EXECUTE_HANDLER");
}
/* ... */
}
void unmask_fpsr(void) {
unsigned int u;
unsigned int control_word;
_controlfp_s(&control_word, 0, 0);
u = control_word & ~(_EM_INVALID \| _EM_DENORMAL \| _EM_ZERODIVIDE | _EM_OVERFLOW | _EM_UNDERFLOW | _EM_INEXACT);
_controlfp_s( &control_word, u, _MCW_EM);
return ;
}
int fpieee_handler(_FPIEEE_RECORD *ieee) {
/* ... */
switch (ieee->RoundingMode) {
case _FpRoundNearest:
/* ... */
break;
/* Other RMs include _FpRoundMinusInfinity, _FpRoundPlusInfinity, _FpRoundChopped */
/* ... */
}
switch (ieee->Precision) {
case _FpPrecision24:
/* ... */
break;
/* Other Ps include _FpPrecision53*/
/* ... */
}
switch (ieee->Operation) {
case _FpCodeAdd:
/* ... */
break;
/* Other Ops include _FpCodeSubtract, _FpCodeMultiply, _FpCodeDivide, _FpCodeSquareRoot, _FpCodeCompare, _FpCodeConvert, _FpCodeConvertTrunc */
/* ... */
}
/* process the bitmap ieee->Cause */
/* process the bitmap ieee->Enable */
/* process the bitmap ieee->Status */
/* process the Operand ieee->Operand1, evaluate format and Value */
/* process the Operand ieee->Operand2, evaluate format and Value */
/* process the Result ieee->Result, evaluate format and Value */
/* the result should be set according to the operation specified in ieee->Cause and the result format as specified in ieee->Result */
/* the Result set is based on the */
/* ... */
}
|
...