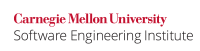
Most implementations of C uses use the IEEE 754 standard for floating point representation. In this representation floats are encoded using 1 sign bit, 8 exponent bits and 23 mantissa bits. Doubles are encoded and used exactly the same way except they use 1 sign bit, 11 exponent bits, and 52 mantissa bits.These bits encode the values of s, the sign; M, the significand; and E, the exponent. Floating point numbers are then calculated as (-1)^s * M * 2^E.
...
This code attempts to reduce a floating point number to a denormalized value and then restore the value. This operation is very imprecise. FLP02-C
Code Block | ||
---|---|---|
| ||
#include <stdio.h>
int main() {
float x = 1/3.0;
printf("Original : %e\n", x);
x = x * 7e-45;
printf("Denormalized? : %e\n", x);
x = x / 7e-45;
printf("Restored : %e\n", x);
}
|
...