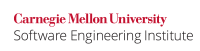
...
The following code generates an ID with a numeric part produced by calling the rand()
function. The IDs produced are predictable and have limited randomness.
Code Block | ||
---|---|---|
| ||
enum {len = 12}; char id[len]; /* id will hold the ID, starting with * the characters "ID" followed by a * random integer */ int r; int num; /* ... */ r = rand(); /* generate a random integer */ num = snprintf(id, len, "ID%-d", r); /* generate the ID */ /* ... */ |
...
A better pseudorandom number generator is the random()
function. While the low dozen bits generated by rand()
go through a cyclic pattern, all the bits generated by random()
are usable.
Code Block | ||
---|---|---|
| ||
enum {len = 12}; char id[len]; /* id will hold the ID, starting with * the characters "ID" followed by a * random integer */ int r; int num; /* ... */ time_t now = time(NULL); if (now == (time_t) -1) { /* handle error */ } srandom(now); /* seed the PRNG with the current time */ /* ... */ r = random(); /* generate a random integer */ num = snprintf(id, len, "ID%-d", r); /* generate the ID */ /* ... */ |
...
Although not specified by POSIX, arc4random()
is an option on systems that support it. From the arc4random(3)
manual page:
arc4random()
fits into a middle ground not covered by other subsystems such as the strong, slow, and resource expensive random devices described inrandom(4)
versus the fast but poor quality interfaces described inrand(3)
,random(3)
, anddrand48(3)
.
...
Wiki Markup If an application has access to a good random source, it can fill the {{pbBuffer}} buffer with some random data before calling {{CryptGenRandom()}}. The CSP \[cryptographic service provider\] then uses this data to further randomize its internal seed. It is acceptable to omit the step of initializing the {{pbBuffer}} buffer before calling {{CryptGenRandom()}}.
Code Block | ||
---|---|---|
| ||
#include<Wincrypt.h>
HCRYPTPROV hCryptProv;
union {
BYTE bs[sizeof(long int)];
long int li;
} rand_buf;
if (!CryptGenRandom(hCryptProv, sizeof(rand_buf), &rand_buf) {
/* Handle error */
} else {
printf("Random number: %ld\n", rand_buf.li);
}
|
...
Wiki Markup |
---|
\[[ISO/IEC 9899:1999|AA. C References#ISO/IEC 9899-1999]\] Section 7.20.2.1, "The rand function" \[[MITRE 07|AA. C References#MITRE 07]\] [CWE ID 327|http://cwe.mitre.org/data/definitions/327.html], "Use of a Broken or Risky Cryptographic Algorithm," [CWE ID 330|http://cwe.mitre.org/data/definitions/330.html], "Use of Insufficiently Random Values" \[[MSDN|AA. C References#MSDN]\] "[CryptGenRandom Function|http://msdn.microsoft.com/en-us/library/aa379942.aspx]" |
...
49. Miscellaneous (MSC) MSC31-C. Ensure that return values are compared against the proper type