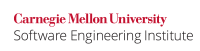
...
Opening
...
and
...
closing
...
braces
...
for
...
if
...
,
...
for
...
,
...
or
...
while
...
statements
...
should
...
always
...
be
...
used,
...
even
...
if
...
said
...
statement
...
has
...
only
...
a
...
single
...
body
...
line.
...
Braces
...
help
...
improve
...
the
...
uniformity,
...
and
...
therefore
...
readability
...
of
...
code.
...
More
...
importantly,
...
when
...
inserting
...
an
...
additional
...
statement
...
in
...
a
...
body
...
containing
...
only
...
a
...
single
...
line,
...
it
...
is
...
easy
...
to
...
forget
...
to
...
add
...
braces
...
when
...
the
...
indentation
...
tends
...
to
...
give
...
a
...
strong
...
(but
...
probably
...
misleading)
...
guide
...
to
...
the
...
structure.
...
Noncompliant
...
Code
...
Example
...
This
...
noncompliant
...
code
...
example
...
uses
...
an
...
if-else
...
statement
...
without
...
braces
...
to
...
authenticate
...
a
...
user.
Code Block | ||||
---|---|---|---|---|
| =
| |||
} int login; if (invalid_login()) login = 0; else login = 1; {code} |
The
...
programmer
...
adds
...
a
...
debugging
...
statement
...
to
...
determine
...
when
...
the
...
login
...
is
...
valid,
...
but
...
forgets
...
to
...
add
...
opening
...
and
...
closing
...
braces.
Code Block | ||||
---|---|---|---|---|
| =
| |||
} int login; if (invalid_login()) login = 0; else printf("Login is valid\n"); /* debugging line added here */ login = 1; {code} |
Due
...
to
...
the
...
indentation
...
of
...
the
...
code,
...
it
...
is
...
difficult
...
to
...
tell
...
that
...
the
...
code
...
is
...
not
...
functioning
...
as
...
intended
...
by
...
the
...
programmer,
...
leading
...
to
...
a
...
possible
...
security
...
breach.
Compliant Solution
Opening and closing braces are used even when the body is a single statement.
Code Block | ||
---|---|---|
| ||
h3. Compliant Code Example
Opening and closing braces are used even when the body is a single statement.
{code:bgColor=#ffcccc}
int login;
if (invalid_login()) {
login = 0;
} else {
login = 1;
}
|
Noncompliant Code Example
When you have an if-else
statement nested in another if
statement, always put braces around the if-else
.
This noncompliant code example does not use braces.
Code Block | ||
---|---|---|
| ||
{code}
h3. Noncompliant Code Example
When you have an {{if-else}} statement nested in another {{if}} statement, always put braces around the {{if-else}}.
This noncompliant code example does not use braces.
{code:bgColor=#ffcccc}
if (a)
if (b)
win();
else
lose();
|
Compliant Solution
Code Block | ||
---|---|---|
| ||
{code} h3. Compliant Code Example {code:bgColor=#ffcccc} if (a) { if (b) { win(); } else { lose(); } } |
Noncompliant Code Example
Macros can be used to execute a sequence of multiple statements as group.
In the event that multiple statements in a macro is not bound in a do-while loop (see PRE10-C. Wrap multi-statement macros in a do-while loop), an if statement with opening and closing braces will still ensure that all statements of the macro are properly executed.
Code Block | ||
---|---|---|
| ||
/*
* Swaps two values.
* Requires tmp variable to be defined.
*/
#define SWAP(x, y) \
tmp = x; \
x = y; \
y = tmp
|
This macro will expand correctly in a normal sequence of statements, but not as the then-clause in an if statement:
Code Block | ||
---|---|---|
| ||
int x, y, z, tmp; if (z == 0) SWAP( x, y); |
This will expand to:
Code Block | ||
---|---|---|
| ||
int x, y, z, tmp;
if (z == 0)
tmp = x;
x = y;
y = tmp;
|
Compliant Solution
Given an if
statement bounded with opening and closing braces, the macro would expand as intended.
Code Block | ||
---|---|---|
| ||
int x, y, z, tmp;
if (z == 0) {
tmp = x;
x = y;
y = tmp;
}