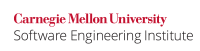
...
Code Block | ||
---|---|---|
| ||
int func(int, int, int); /* ... */ func(1, 2); /* ... */ int func(int one, int two, int three){ printf("%d %d %d", one, two, three); return 1; } |
Non-Compliant Code Example: (function pointers)
If a function pointer is set to refer to an incompatible function, invoking that function via the pointer may cause unexpected data to be taken from the process stack. As a result, unexpected data may be accessed by the called function.
Setting fn_ptr
to point to add()
could result in an unexpected value being used as parameter z
in function add()
.
Code Block | ||
---|---|---|
| ||
int add(int x, int y, int z) {
return x + y + z;
}
int main(int argc, char *argv[]) {
int (*fn_ptr) (int, int) ;
int res;
fn_ptr = add;
res = fn_ptr(2, 3); /* incorrect */
/* ... */
return 0;
}
|
Compliant Solution: (function pointers)
To correct this example, the declaration of fn_ptr
is changed to accept three arguments.
Code Block | ||
---|---|---|
| ||
int add(int x, int y, int z) {
return x + y + z;
}
int main(int argc, char *argv[]) {
int (*fn_ptr) (int, int, int) ;
int res;
fn_ptr = add;
res = fn_ptr(2, 3, 4);
/* ... */
return 0;
}
|
Risk Assessment
Failing to include type information for function declarators can result in unexpected or unintended program behavior.
...