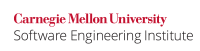
...
Code Block |
---|
void foo(int x) { x = 3; /* persists only lastsuntil untilthe endfunction ofexits function */ /* ... */ } |
Pointers behave in a similar fashion. A function may change a pointer to reference a different object, or NULL, yet that change is discarded once the function exits. Consequently, declaring a pointer as const
is unnecessary.
Code Block |
---|
void foo(int *x) { x = NULL; /* persists only lastsuntil untilthe endfunction ofexits function */ /* ... */ } |
Noncompliant Code Example
...
Code Block | ||
---|---|---|
| ||
char *strcat_nc(char *s1, char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[9] = "str3"; const char str4[9] = "str4"; strcat_nc(str3, str2); /* Compiler warns that str2 is const */ strcat_nc(str1, str3); /* Attempts to overwrite string literal! */ strcat_nc(str4, str3); /* Compiler warns that str4 is const */ |
...
In the first strcat_nc()
call, the compiler will generate generates a warning about attempting to cast away const on str2
. This is a good warning, as because strcat_nc()
does not modify its second argument, yet fails to declare it const
.
In the second strcat_nc()
call, the compiler will happily compile compiles the code with no warnings, but the resulting code will attempt to modify the "str1"
literal, which may be impossible; the literal may not be defined in the heap. This violates STR05-C. Use pointers to const when referring to string literals and STR30-C. Do not attempt to modify string literals.
In the final strcat_nc()
call, the compiler generates a warning about attempting to cast away const
on str4
. This is a valid warning.
...
Code Block | ||
---|---|---|
| ||
char *strcat(char *s1, const char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[9] = "str3"; const char str4[9] = "str4"; strcat(str3, str2); /* Args reversed to prevent overwriting string literal */ strcat(str3, str1); strcat(str4, str3); /* Compiler warns that str4 is const */ |
The const
-qualification of the second argument s2
eliminates the spurious warning in the initial invocation, but maintains the valid warning on the final invocation in which a const
-qualified object is passed as the first argument (which can change). Finally, the middle strcat()
invocation is now valid, as str1
str3
is a valid destination string , as the string exists on the stack and may be safely modified.
...