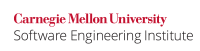
...
Code Block |
---|
extern char **environ; /* ... */ int main(int argc, const char const *argv[], const char const *envp[]) { printf("environ: %p\n", environ); printf("envp: %p\n", envp); setenv("MY_NEW_VAR", "new_value", 1); puts("--Added MY_NEW_VAR--"); printf("environ: %p\n", environ); printf("envp: %p\n", envp); } |
...
It is evident from these results that the environment has been relocated as a result of the call to setenv()
.
...
Noncompliant Code Example (POSIX)
Wiki Markup |
---|
After a call to the POSIX {{setenv()}} function, or other function that modifies the environment, the {{envp}} pointer may no longer reference the environment. POSIX states that \[[Open Group 04|AA. C References#Open Group 04]\] |
...
Code Block | ||
---|---|---|
| ||
int main(int argc, const char const *argv[], const char const *envp[]) { size_t i; if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle Error */ } if (envp != NULL) { for (i = 0; envp[i] != NULL; i++) { if (puts(envp[i]) == EOF) { /* Handle Error */ } } } return 0; } |
...
Code Block | ||
---|---|---|
| ||
extern char **environ; /* ... */ int main(int argc, const char const *argv[]) { size_t i; if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle Error */ } if (environ != NULL) { for (i = 0; environ[i] != NULL; i++) { if (puts(environ[i]) == EOF) { /* Handle Error */ } } } return 0; } |
...
Noncompliant Code Example (Windows)
After a call to the Windows _putenv_s()
function, or other function that modifies the environment, the envp
pointer may no longer reference the environment.
...
Code Block | ||
---|---|---|
| ||
int main(int argc, const char const *argv[], const char const *envp[]) { size_t i; if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle Error */ } if (envp != NULL) { for (i = 0; envp[i] != NULL; i++) { if (puts(envp[i]) == EOF) { /* Handle Error */ } } } return 0; } |
...
Code Block | ||
---|---|---|
| ||
_CRTIMP extern char **_environ; /* ... */ int main(int argc, const char const *argv[]) { size_t i; if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle Error */ } if (_environ != NULL) { for (i = 0; _environ[i] != NULL; i++) { if (puts(_environ[i]) == EOF) { /* Handle Error */ } } } return 0; } |
...