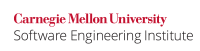
...
In C, function arguments are passed by value rather than by reference. While a function may change the values passed in, these changed values are discarded once the function returns. For this reason, many programmers assume a function will not change its arguments, and declaring the functions function's parameters as const
is unnecessary.
...
Unlike passed-by-value arguments and pointers, pointed-to values are a concern. A function may modify a value referenced by a pointer argument, leading to a side effect which persists even after the function exits. Modification of the pointed-to value is not diagnosed by the complier, which assumes this was the intended behavior.
Code Block | ||
---|---|---|
| ||
void foo(int *x) { if (x != NULL) { *x = 3; /* visible outside function */ } /* ... */ } |
...
This noncompliant code example , defines a fictional version of the standard strcat()
function called strcat_nc()
. This function differs from strcat()
in that the second argument is not const
-qualified.
Code Block | ||
---|---|---|
| ||
char *strcat_nc(char *s1, char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[9] = "str3"; const char str4[9] = "str4"; strcat_nc(str3, str2); /* Compiler warns that str2 is const */ strcat_nc(str1, str3); /* Attempts to overwrite string literal! */ strcat_nc(str4, str3); /* Compiler warns that str4 is const */ |
The function would behave behaves the same as strcat()
, but the compiler generates warnings in incorrect locations , and fails to generate them in correct locations.
...
Code Block | ||
---|---|---|
| ||
char *strcat(char *s1, const char *s2); char *str1 = "str1"; const char *str2 = "str2"; char str3[9] = "str3"; const char str4[9] = "str4"; strcat(str3, str2); /* Args reversed to prevent overwriting string literal */ strcat(str3, str1); strcat(str4, str3); /* Compiler warns that str4 is const */ |
The const
-\qualification of the second argument s2
eliminates the spurious warning in the initial invocation , but maintains the valid warning on the final invocation in which a const
-qualified object is passed as the first argument (which can change). Finally, the middle strcat()
invocation is now valid, as str3
is a valid destination string and may be safely modified.
...