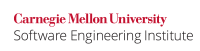
...
Code Block |
---|
size_t count; /* must be nonzero */ char *to; /* output destination */ char *from; /* Points to count bytes to copy */ do { *to = *from++; /* Note that the ''"to''" pointer is NOT incremented */ } while (--count > 0); |
...
Code Block | ||||
---|---|---|---|---|
| ||||
int n = (count + 7) / 8; switch (count % 8) { case 0: do { *to = *from++; case 7: *to = *from++; case 6: *to = *from++; case 5: *to = *from++; case 4: *to = *from++; case 3: *to = *from++; case 2: *to = *from++; case 1: *to = *from++; } while (--n > 0); } |
In this code, the first iteration of the loop is subject to the switch
statement, so it performs count % 8
assignments. Each subsequent iteration of the loop performs 8 assignments. (Being outside the loop, the switch
statement is ignored.) Consequently, this code performs count
assignments, but only n
comparisons, so it is usually faster.
...
Compliant Code Example (Duff's Device)
This is an alternate implemention implementation of Duff's device, which separates the switch
statement and loop.
Code Block | ||||
---|---|---|---|---|
| ||||
int n = (count + 7) / 8; switch (count % 8) { case 0: *to = *from++; /* fall through */ case 7: *to = *from++; /* fall through */ case 6: *to = *from++; /* fall through */ case 5: *to = *from++; /* fall through */ case 4: *to = *from++; /* fall through */ case 3: *to = *from++; /* fall through */ case 2: *to = *from++; /* fall through */ case 1: *to = *from++; /* fall through */ } while (--n > 0) { *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; } |
...
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC20-C | medium | probable | medium | P8 | L2 |
Related Guidelines
...
Bibliography
...
] | Section 6.8.6.1, "The goto statement" |
MISRA Rule 15.1
...