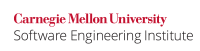
Copying data to a buffer that is not large enough to hold that data results in a buffer overflow. While not limited to null-terminated byte strings (NTBS), buffer overflows often occurs when manipulating NTBS data. To prevent such errors, limit copies either through truncation (see STR03-C. Do not inadvertently truncate a null-terminated byte string) or, preferably, ensure that the destination is of sufficient size to hold the character data to be copied and the null-termination character.
...
Noncompliant Code Example (off-by-one error)
Wiki Markup |
---|
This non-compliantnoncompliant code example demonstrates what is commonly referred to as an _off-by-one_ error \[[Dowd 06|AA. C References#Dowd 06]\]. The loop copies data from {{src}} to {{dest}}. However, the null terminator may incorrectly be written one byte past the end of {{dest}}. The flaw exists because the loop does not account for the null-termination character that must be appended to {{dest}}. |
...
Code Block | ||
---|---|---|
| ||
char dest[ARRAY_SIZE]; char src[ARRAY_SIZE]; size_t i; /* ... */ for (i=0; src[i] && (i < sizeof(dest)-1); i++) { dest[i] = src[i]; } dest[i] = '\0'; /* ... */ |
...
Noncompliant Code Example (argv
)
Arguments read from the command line are stored in process memory. The function main()
, called at program startup, is typically declared as follows when the program accepts command-line arguments:
...
Wiki Markup |
---|
The parameters {{argc}} and {{argv}} and the strings pointed to by the {{argv}} array are not modifiable by the program and retain their last-stored values between program startup and program termination. This requires that a copy of these parameters be made before the strings can be modified. Vulnerabilities can occur when inadequate space is allocated to copy a command-line argument. In this non-compliantnoncompliant code example, the contents of {{argv\[0\]}} can be manipulated by an attacker to cause a buffer overflow: |
...
Code Block | ||
---|---|---|
| ||
int main(int argc, char *argv[]) { /* ... */ const char const *progname = argv[0]; size_t prog_size; /* ... */ } |
...
Noncompliant Code Example (getenv()
)
The getenv()
function searches an environment list, provided by the host environment, for a string that matches the string pointed to by name. The set of environment names and the method for altering the environment list are implementation-defined. Environment variables can be arbitrarily large, and copying them into fixed-length arrays without first determining the size and allocating adequate storage can result in a buffer overflow.
...