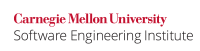
...
In this noncompliant code example, it is possible that the the f_imp()
function is given a valid iterator but that the iterator is not within the (correct) ending iterator e
for a container, and b
is an interator to the same container. Unfortunately, it is possible that b
is not within the valid range of its container. For instance, if f()
were called with iterators obtained from an empty container, the end()
iterator could be if the container were empty, b
would equal e
and be improperly dereferenced.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <iterator> template <typename ForwardIterator> void f_imp(ForwardIterator b, ForwardIterator e, int val, std::forward_iterator_tag) { do { *b++ = val; } while (b != e); } template <typename ForwardIterator> void f(ForwardIterator b, ForwardIterator e, int val) { typename std::iterator_traits<ForwardIterator>::iterator_category cat; f_imp(b, e, val, cat); } |
...
This compliant solution tests for iterator validity before attempting to dereference the forward iterator b
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <iterator> template <typename ForwardIterator> void f_imp(ForwardIterator b, ForwardIterator e, int val, std::forward_iterator_tag) { while (b != e) { *b++ = val; } } template <typename ForwardIterator> void f(ForwardIterator b, ForwardIterator e, int val) { typename std::iterator_traits<ForwardIterator>::iterator_category cat; f_imp(b, e, val, cat); } |
...