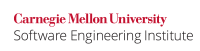
...
In this noncompliant code example, the SomeClass
destructor attempts to handle exceptions thrown from the destructor of the bad_member
subobject by absorbing them.we presume the existence of a Bad
class with a destructor that can throw, and although it violates this rule, we cannot modify it:
Code Block | ||||
---|---|---|---|---|
| ||||
// Assume that this class is provided by a 3rd party and it is not something // that can be modified by the user. class Bad { ~Bad() noexcept(false); }; |
In order to safely use the Bad
class, the SomeClass
destructor attempts to handle exceptions thrown from the Bad
destructor by absorbing them:
Code Block | ||||
---|---|---|---|---|
| ||||
class SomeClass {
Bad bad_member;
public:
~SomeClass()
try {
// ...
} catch(...) {
// Handle the exception thrown from the Bad destructor.
}
};
|
...
A destructor should perform the same way whether or not there is an active exception. Typically, this means that it should invoke only operations that do not throw exceptions, or it should handle all exceptions and not rethrow them (even implicitly). This compliant solution differs from the previous noncompliant code example by having an explicit return
statement in the SomeClass
destructor. This statement prevents control from reaching the end of the exception handler. Consequently, this handler will catch the exception thrown by Bad::~Bad()
when bad_member
is destroyed, and it . It will also catch any exceptions thrown within the compound statement of the function-try-block, but the SomeClass
destructor will not terminate by throwing an exception.
Code Block | ||||
---|---|---|---|---|
| ||||
// Assume that this class is provided by a 3rd party and it is not something
// that can be modified by the user.
class Bad {
~Bad() noexcept(false);
};
class SomeClass {
Bad bad_member;
public:
~SomeClass()
try {
// ...
} catch(...) {
// Catch exceptions thrown from noncompliant destructors of
// member objects or base class subobjects.
// NOTE: Flowing off the end of a destructor function-try-block causes
// the caught exception to be implicitly rethrown, but an explicit
// return statement will prevent that from happening.
return;
}
}; |
...