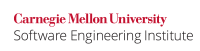
...
The above code examples perform the same when working with vectors.
Code Block | ||
---|---|---|
| ||
enum { TABLESIZE = 100 };
vector<int> table;
int insert_in_table(int pos, int value){
if (pos >= table.size()) {
return -1;
}
table[pos] = value;
return 0;
}
|
The function performs a range check to ensure that pos
does not exceed the upper bound of the array but fails to check the lower bound for table
. Because pos
has been declared as a (signed) int
, this parameter can easily assume a negative value, resulting in a write outside the bounds of the memory referenced by table
.
Compliant Solution (
...
Vectors, size_t
)
In this compliant solution, the parameter pos
is declared as size_t
, which prevents passing of negative arguments (see INT01-CPP. Use rsize_t or size_t for all integer values representing the size of an object).
Code Block | ||
---|---|---|
| ||
enum { TABLESIZE = 100 }; vector<int> table; int insert_in_table(size_t pos, int value){ if (pos >= table.size()) { return -1; } table[pos] = value; return 0; } |
Compliant Solution (Vectors, at()
)
In this compliant solution, access to the vector is accomplished with the at()
method. This method provides bounds checking, throwing an out_of_range
exception if pos
is not a valid index value.
Code Block | ||
---|---|---|
| ||
vector<int> table;
int insert_in_table(size_t pos, int value){
table.at(pos) = value;
return 0;
}
|
Risk Assessment
...